[1] http://docs.blackfin.uclinux.org/doku.php?id=linux-kernel:programming
[2] Understanding the Linux Kernel, 3rd Edition By Daniel P. Bovet, Marco Cesati Publisher: O'Reilly Pub Date: November 2005 ISBN: 0-596-00565-2 Pages: 942
[3] http://publib.boulder.ibm.com/infocenter/iseries/v5r3/index.jsp?topic=/apis/apiexusmem.htm
KERNEL THREAD
--------------------
The only difference between a kernel thread and a user thread is the fact that the kernel thread has no user task memory mapping. Like other user processes it shares the kernel address space but the thread operates only in kernel space with kernel privilages.
A thread is normally designed as a continous loop that calls some wait or scheduling function to stop it consuming 100% of the CPU.
Process creat thread
SPIN LOCK
----------------------------
As a general rule, kernel preemption is disabled in every critical region protected by spin locks. In the case of a uniprocessor system, the locks themselves are useless, and the spin lock primitives just disable or enable the kernel preemption. Please notice that kernel preemption is still enabled during the busy wait phase, thus a process waiting for a spin lock to be released could be replaced by a higher priority process. [2] 5.2.4
SEMAPHORES
-------------------------
Why need semaphore?
In general, safe access to a global variable is ensured by using atomic operations . In the previous example, data corruption is not possible if the two control paths read and decrease V with a single, noninterruptible operation. However, kernels contain many data structures that cannot be accessed with a single operation.
The down( ) method decreases the value of the semaphore. If the new value is less than 0, the method adds the running process to the semaphore list and then blocks (i.e., invokes the scheduler). The up( ) method increases the value of the semaphore and, if its new value is greater than or equal to 0, reactivates one or more processes in the semaphore list. [2] 1.6.5
Each data structure to be protected has its own semaphore, which is initialized to 1. When a kernel control path wishes to access the data structure, it executes the down( ) method on the proper semaphore. If the value of the new semaphore isn't negative, access to the data structure is granted. Otherwise, the process that is executing the kernel control path is added to the semaphore list and blocked.
[2] 1.6.5.3
Linux offers two kinds of semaphores:
Kernel semaphores, which are used by kernel control paths
System V IPC semaphores, which are used by User Mode processes [2] 5.2.8
The semaphore value is positive if the protected resource is available, and 0 if the protected resource is currently not available. A process that wants to access the resource tries to decrease the semaphore value; the kernel, however, blocks the process until the operation on the semaphore yields a positive value. When a process relinquishes a protected resource, it increases its semaphore value; in doing so, any other process waiting for the semaphore is woken up. [2] 19.3.3
int semget(key_t key, int nsems, int semflg);
int semop(int semid, struct sembuf *sops, unsigned nsops);
int semtimedop(int semid, struct sembuf *sops, unsigned nsops,
struct timespec *timeout);
type struct sembuf containing the following members:
unsigned short sem_num; /* semaphore number */
short sem_op; /* semaphore operation */
short sem_flg; /* operation flags */
sem_flg are IPC_NOWAIT and SEM_UNDO
SAMPLES [3]
/* Oprations on semaphore. */
union semun {
int val; /* Value for SETVAL */
struct semid_ds *buf; /* Buffer for IPC_STAT, IPC_SET */
unsigned short *array; /* Array for GETALL, SETALL */
struct seminfo *__buf; /* Buffer for IPC_INFO
(Linux-specific) */
};
Thursday, July 29, 2010
Linux SPI Driver
Ref [1] http://processors.wiki.ti.com/index.php/Omapl137_linux_spi_driver
Ref [2] Mail list about SPI Slave driver development -- http://www.mail-archive.com/spi-devel-general@lists.sourceforge.net/msg00384.html
Ref [3] Spi dev on sourceforge open source project -- http://spi-devel.sourceforge.net/documentation.shtml
Ref [4] Spi dev on blackfin project -- http://docs.blackfin.uclinux.org/doku.php?id=spi
Ref [2] Mail list about SPI Slave driver development -- http://www.mail-archive.com/spi-devel-general@lists.sourceforge.net/msg00384.html
Ref [3] Spi dev on sourceforge open source project -- http://spi-devel.sourceforge.net/documentation.shtml
Ref [4] Spi dev on blackfin project -- http://docs.blackfin.uclinux.org/doku.php?id=spi

What's new in Linux 2.6
A good article addressed the major improvements in Linux Kernel 2.6.xx, which have started to incorporate embedded support into Linux Kernel Main stream development.
What’s new in Linux Kernel 2.6.xxx
http://www.lynuxworks.com/products/whitepapers/linux-2.6.php3
SOME NOTES OF THIS ARTICLE
-----------------------------------------------------
Timeline:
November 2001: Start of 2.5.x development (2.4.15)
October 2002: Feature freeze for 2.6
Release of Linux 2.6.0: Dec-2003
USB 2.0 also makes its debut on Linux 2.6.
Version 2.6 integrates a significant portion of uClinux into the production kernel, bringing microcontroller support into the Linux mainstream.
Linux 2.6 introduces improvements that make it a far more worthy platform than in the past when responsiveness was an issue. The three most significant improvements are:
• Preemption points in the kernel
• An efficient scheduler
• Enhanced synchronization
SOME INTERESTING BACKGROUND INFO ABOUT LINUX TO READ
----------------------------------------------------------------------------------------------------------
Official Linux Kernel Release Web Site:
http://www.kernel.org/
Linux History:
http://en.wikipedia.org/wiki/Linux_history
Linux Kernel:
http://en.wikipedia.org/wiki/Linux_kernel
Estimated cost to redevelop
The cost to redevelop the Linux kernel version 2.6.0 in a traditional proprietary development setting has been estimated to be $612 million USD (€467 million euro) in 2004 prices using the COCOMO man-month estimation model.[55] In 2006, a study funded by the European Union put the redevelopment cost of kernel version 2.6.8 higher, at €882 million euro ($1.14 billion USD).[56]
This topic was revisited in October 2008 by Amanda McPherson, Brian Proffitt and Ron Hale-Evans. Using David A. Wheelers methodology, they estimated redevelopment of the 2.6.25 kernel now costs $1.3 billion (part of a total $10.8 billion to redevelop Fedora 9).[57] Again, Garcia-Garcia and Alonso de Magdaleno from University of Oviedo (Spain) estimate that the value annually added to kernel was about 100 million EUR between 2005 and 2007 and 225 million EUR in 2008, it would cost also more than one billion EUR (about 1.4 billion USD) to develop in European Union. [58]
There are many other well-known maintainers for the Linux kernel beside Torvalds such as Alan Cox and Marcelo Tosatti. Cox maintained version 2.2 of the kernel until it was discontinued at the end of 2003.
Likewise, Tosatti maintained version 2.4 of the kernel until the middle of 2006.
Andrew Morton steers the development and administration of the 2.6 kernel, which was released on 18 December 2003 in its first stable incarnation.
Also the older branches are still constantly improved.
• 25 January 1999 - Linux 2.2.0 was released (1,800,847 lines of code).
• 18 December 1999 - IBM mainframe patches for 2.2.13 were published, allowing Linux to be used on enterprise-class machines.
• 4 January 2001 - Linux 2.4.0 was released (3,377,902 lines of code).
• 17 December 2003 - Linux 2.6.0 was released (5,929,913 lines of code).
• 16 May 2010 - Linux 2.6.34 was released (13,320,934 lines of code).[16]
What’s new in Linux Kernel 2.6.xxx
http://www.lynuxworks.com/products/whitepapers/linux-2.6.php3
SOME NOTES OF THIS ARTICLE
-----------------------------------------------------
Timeline:
November 2001: Start of 2.5.x development (2.4.15)
October 2002: Feature freeze for 2.6
Release of Linux 2.6.0: Dec-2003
USB 2.0 also makes its debut on Linux 2.6.
Version 2.6 integrates a significant portion of uClinux into the production kernel, bringing microcontroller support into the Linux mainstream.
Linux 2.6 introduces improvements that make it a far more worthy platform than in the past when responsiveness was an issue. The three most significant improvements are:
• Preemption points in the kernel
• An efficient scheduler
• Enhanced synchronization
SOME INTERESTING BACKGROUND INFO ABOUT LINUX TO READ
----------------------------------------------------------------------------------------------------------
Official Linux Kernel Release Web Site:
http://www.kernel.org/
Linux History:
http://en.wikipedia.org/wiki/Linux_history
Linux Kernel:
http://en.wikipedia.org/wiki/Linux_kernel
Estimated cost to redevelop
The cost to redevelop the Linux kernel version 2.6.0 in a traditional proprietary development setting has been estimated to be $612 million USD (€467 million euro) in 2004 prices using the COCOMO man-month estimation model.[55] In 2006, a study funded by the European Union put the redevelopment cost of kernel version 2.6.8 higher, at €882 million euro ($1.14 billion USD).[56]
This topic was revisited in October 2008 by Amanda McPherson, Brian Proffitt and Ron Hale-Evans. Using David A. Wheelers methodology, they estimated redevelopment of the 2.6.25 kernel now costs $1.3 billion (part of a total $10.8 billion to redevelop Fedora 9).[57] Again, Garcia-Garcia and Alonso de Magdaleno from University of Oviedo (Spain) estimate that the value annually added to kernel was about 100 million EUR between 2005 and 2007 and 225 million EUR in 2008, it would cost also more than one billion EUR (about 1.4 billion USD) to develop in European Union. [58]
There are many other well-known maintainers for the Linux kernel beside Torvalds such as Alan Cox and Marcelo Tosatti. Cox maintained version 2.2 of the kernel until it was discontinued at the end of 2003.
Likewise, Tosatti maintained version 2.4 of the kernel until the middle of 2006.
Andrew Morton steers the development and administration of the 2.6 kernel, which was released on 18 December 2003 in its first stable incarnation.
Also the older branches are still constantly improved.
• 25 January 1999 - Linux 2.2.0 was released (1,800,847 lines of code).
• 18 December 1999 - IBM mainframe patches for 2.2.13 were published, allowing Linux to be used on enterprise-class machines.
• 4 January 2001 - Linux 2.4.0 was released (3,377,902 lines of code).
• 17 December 2003 - Linux 2.6.0 was released (5,929,913 lines of code).
• 16 May 2010 - Linux 2.6.34 was released (13,320,934 lines of code).[16]
Sunday, July 25, 2010
Make file in GCC
missing separator. Stop.
-------------------------------
From: http://www.delorie.com/djgpp/v2faq/faq22_17.html
Q: When I invoke Make, it refuses to do anything and prints a cryptic message: "makefile:10: *** missing separator. Stop." Now what kind of excuse is that?
A: Unlike most other DOS Make programs which accept any whitespace character at the beginning of a command in a rule, GNU Make insists that every such line begins with a TAB. (Most other Unix Make programs also require TABs, and the Posix standard requires it as well.) Make sure that the line whose number is printed in the error message (in this case, line 10) begins with a TAB.
Hello world sample using make file
-------------------------------------
[q.yang@localhost Gsn_HelloEclipse]$ pwd
/home/q.yang/lpc3250/ltib-qs/rootfs/home/user/Gsn_HelloEclipse
[q.yang@localhost Gsn_HelloEclipse]$ tree
[q.yang@localhost Gsn_HelloEclipse]$ cat Makefile
[q.yang@localhost Gsn_HelloEclipse]$ cat ./sources/Makefile
[q.yang@localhost Gsn_HelloEclipse]$ cat ./sources/hellocpp.cpp
[q.yang@localhost Gsn_HelloEclipse]$ make
[q.yang@localhost Gsn_HelloEclipse]$ tree
[q.yang@localhost Gsn_HelloEclipse]$ make clean
[q.yang@localhost Gsn_HelloEclipse]$ tree
-------------------------------
From: http://www.delorie.com/djgpp/v2faq/faq22_17.html
Q: When I invoke Make, it refuses to do anything and prints a cryptic message: "makefile:10: *** missing separator. Stop." Now what kind of excuse is that?
A: Unlike most other DOS Make programs which accept any whitespace character at the beginning of a command in a rule, GNU Make insists that every such line begins with a TAB. (Most other Unix Make programs also require TABs, and the Posix standard requires it as well.) Make sure that the line whose number is printed in the error message (in this case, line 10) begins with a TAB.
Hello world sample using make file
-------------------------------------
[q.yang@localhost Gsn_HelloEclipse]$ pwd
/home/q.yang/lpc3250/ltib-qs/rootfs/home/user/Gsn_HelloEclipse
[q.yang@localhost Gsn_HelloEclipse]$ tree
.
|-- Makefile
|-- debug
|-- include
`-- sources
|-- Makefile
`-- hellocpp.cpp
[q.yang@localhost Gsn_HelloEclipse]$ cat Makefile
all:
make -C sources
mv ./sources/*.o ./debug
mv ./sources/hellocpp ./debug
clean:
rm -f ./debug/*.o
rm -f ./debug/hellocpp
[q.yang@localhost Gsn_HelloEclipse]$ cat ./sources/Makefile
OBJS = hellocpp.o
CC = arm-none-linux-gnueabi-g++
DEBUG = -g
CFLAGS = -Wall -c $(DEBUG)
LFLAGS = -Wall $(DEBUG)
INCL = -I. -I../ -I../Include
all:hellocpp
hellocpp: $(OBJS)
$(CC) -o hellocpp $(OBJS)
%.o: %.cpp
$(CC) -c $(CFLAGS) $(INCL) $< -o $@
[q.yang@localhost Gsn_HelloEclipse]$ cat ./sources/hellocpp.cpp
#include
using namespace std;
int main()
{
cout << "Hello world!" << endl;
cout << "Hello there. First GsnCpp from Eclipse IDE.\n";
return 0;
}
[q.yang@localhost Gsn_HelloEclipse]$ make
make -C sources
make[1]: Entering directory `/home/q.yang/lpc3250/ltib-qs/rootfs/home/user/Gsn_HelloEclipse/sources'
arm-none-linux-gnueabi-g++ -c -Wall -c -g -I. -I../ -I../Include hellocpp.cpp -o hellocpp.o
arm-none-linux-gnueabi-g++ -o hellocpp hellocpp.o
make[1]: Leaving directory `/home/q.yang/lpc3250/ltib-qs/rootfs/home/user/Gsn_HelloEclipse/sources'
mv ./sources/*.o ./debug
mv ./sources/hellocpp ./debug
[q.yang@localhost Gsn_HelloEclipse]$ tree
.
|-- Makefile
|-- debug
| |-- hellocpp
| `-- hellocpp.o
|-- include
`-- sources
|-- Makefile
`-- hellocpp.cpp
[q.yang@localhost Gsn_HelloEclipse]$ make clean
rm -f ./debug/*.o
rm -f ./debug/hellocpp
[q.yang@localhost Gsn_HelloEclipse]$ tree
.
|-- Makefile
|-- debug
|-- include
`-- sources
|-- Makefile
`-- hellocpp.cpp
Thursday, July 22, 2010
Installation of Eclipse CDT IDE in Fedora 9.
Ref [1] http://infocenter.arm.com/help/index.jsp?topic=/com.arm.doc.dai0201a/index.html Debugging Linux Application using Eclipse+GDB
1. Download latest Eclipse CDT IDE.
--------------------------------------------
From:
http://www.eclipse.org/downloads/download.php?file=/technology/epp/downloads/release/helios/R/eclipse-cpp-helios-linux-gtk.tar.gz
[q.yang@localhost Download]$ ll
-rwxr--r-- 1 q.yang q.yang 91574228 2010-07-23 14:25 eclipse-cpp-helios-linux-gtk.tar.gz
3. Installation Eclipse CDT (IDE 3.6.0 CDT 7.0.0)
----------------------------------------------------
[q.yang@localhost ~]$ mkdir EclipseCDT
[q.yang@localhost EclipseCDT]$ tar zxf ~/Download/eclipse-cpp-helios-linux-gtk.tar.gz

4. Start first Eclipse Project
------------------------------------------
[q.yang@localhost eclipse]$./eclipse
Then set work space storage folder
/home/q.yang/EclipseCDT/qywkspace
Add /home/q.yang/EclipseCDT/eclipse/ into $PATH so that command line '$eclipse' will open eclipse IDE.
5. Create Make file based C/C++ application project
---------------------------------------------------
Not makefile will be generated by eclipse CDT. Good for import existing makefile based source codes and projects. Only use eclipse as a debugger mainly and trigger 'make'.
New-->C++ project
Then give the project name 'GsnProjEclipseHelloCpp'.
And uncheck 'use default location', browse to choose Root directory of source codes '/home/q.yang/lpc3250/ltib-qs/rootfs/home/user/GsnHelloCppRoot'
As shown in this screen shot.

After creating 'Makefile project', attached is the project view screen shot:

6. Set debug configuration
-----------------------------------------------------
In C/C++ editing view, right click project, choose 'Debug As' -> 'Debug Configurations ...'
Create button to new a debug configuration, rename this new debug configuration as 'GsnProjEclipseDebugConfig'.
Then click browse to choose C/C++ application 'hellocpp', which is the binary executable program generated by makefile.
As shown in this screen shot:

Set up GDB debugger (from LTIB tool chain) and GDB command file as shown in the screen shot:

Set up Debugger Options -> Shared Libraries as shown in this screen shot (LTIB lib)

Set up Debugger Options -> Connection as shown in this screen shot (via Ethernet to target board phytec 3250)

7. Done. You would be able to do single step debugging now.
--------------------------------------------------------------
As shown in this screen shot:
[root@GsnCommsModule debug]# gdbserver 127.0.0.1:2000 hellocpp
Process hellocpp created; pid = 501
Listening on port 2000
Remote debugging from host 10.10.20.70
Hello world!
Hello there. First GsnCpp from Eclipse IDE.
Child exited with retcode = 0
Child exited with status 0
GDBserver exiting
1. Download latest Eclipse CDT IDE.
--------------------------------------------
From:
http://www.eclipse.org/downloads/download.php?file=/technology/epp/downloads/release/helios/R/eclipse-cpp-helios-linux-gtk.tar.gz
[q.yang@localhost Download]$ ll
-rwxr--r-- 1 q.yang q.yang 91574228 2010-07-23 14:25 eclipse-cpp-helios-linux-gtk.tar.gz
3. Installation Eclipse CDT (IDE 3.6.0 CDT 7.0.0)
----------------------------------------------------
[q.yang@localhost ~]$ mkdir EclipseCDT
[q.yang@localhost EclipseCDT]$ tar zxf ~/Download/eclipse-cpp-helios-linux-gtk.tar.gz
4. Start first Eclipse Project
------------------------------------------
[q.yang@localhost eclipse]$./eclipse
Then set work space storage folder
/home/q.yang/EclipseCDT/qywkspace
Add /home/q.yang/EclipseCDT/eclipse/ into $PATH so that command line '$eclipse' will open eclipse IDE.
5. Create Make file based C/C++ application project
---------------------------------------------------
Not makefile will be generated by eclipse CDT. Good for import existing makefile based source codes and projects. Only use eclipse as a debugger mainly and trigger 'make'.
New-->C++ project
Then give the project name 'GsnProjEclipseHelloCpp'.
And uncheck 'use default location', browse to choose Root directory of source codes '/home/q.yang/lpc3250/ltib-qs/rootfs/home/user/GsnHelloCppRoot'
As shown in this screen shot.
After creating 'Makefile project', attached is the project view screen shot:
6. Set debug configuration
-----------------------------------------------------
In C/C++ editing view, right click project, choose 'Debug As' -> 'Debug Configurations ...'
Create button to new a debug configuration, rename this new debug configuration as 'GsnProjEclipseDebugConfig'.
Then click browse to choose C/C++ application 'hellocpp', which is the binary executable program generated by makefile.
As shown in this screen shot:
Set up GDB debugger (from LTIB tool chain) and GDB command file as shown in the screen shot:
[q.yang@localhost qywkspace]$ pwd
/home/q.yang/EclipseCDT/qywkspace
[q.yang@localhost qywkspace]$ ll
total 16
-rw-rw-r-- 1 q.yang q.yang 145 2010-07-26 12:28 eclipseGdbCmdFile
drwxrwxr-x 4 q.yang q.yang 4096 2010-07-26 14:20 Gsn_HelloCpp_Eclipse
[q.yang@localhost qywkspace]$ cat eclipseGdbCmdFile
set remotetimeout 0
set solib-absolute-prefix /opt/freescale/usr/local/gcc-4.1.2-glibc-2.5-nptl-3/arm-none-linux-gnueabi/arm-none-linux-gnueabi/
Set up Debugger Options -> Shared Libraries as shown in this screen shot (LTIB lib)
Set up Debugger Options -> Connection as shown in this screen shot (via Ethernet to target board phytec 3250)
7. Done. You would be able to do single step debugging now.
--------------------------------------------------------------
As shown in this screen shot:
[root@GsnCommsModule debug]# gdbserver 127.0.0.1:2000 hellocpp
Process hellocpp created; pid = 501
Listening on port 2000
Remote debugging from host 10.10.20.70
Hello world!
Hello there. First GsnCpp from Eclipse IDE.
Child exited with retcode = 0
Child exited with status 0
GDBserver exiting
Compiler Linker Libraries for Embedded Linux
[1] Kernel Org about Libc
[2] GNU C Library - GLIBC
From libc.info and Coreutils.info
-----------------------------------
unlink,
rename,
if (stat("/dev/ttyUSB3",&buf)==0) {
//3G modem present
Misc::debug_print((GSN_S8*)"3G modem detected",GSN_LOG_INFO);
Misc::debug_print((GSN_S8*)"Killing pppd (if already running)",GSN_LOG_INFO);
if (system("killall pppd")==0) {
sleep(10);
GPIO_CMM_LNK_OFF;
}
rc = pthread_create(&pppthread, NULL, runpppd, &threadarg);
if (rc == 0) {
Misc::debug_print((GSN_S8*)"ppp thread created, sleeping to allow connection",GSN_LOG_INFO);
sleep(30);
} else {
Misc::debug_print((GSN_S8*)"failed to create ppp thread\n",GSN_LOG_INFO);
exit(1);
}
if (CommsLinkSessionMgr::isPPPConnectionAvailabe()) {
GPIO_CMM_LNK_ON;
} else {
GPIO_CMM_LNK_OFF;
}
}
1. Available Libraries.
-------------------------------------
A. Glibc
From: http://en.wikipedia.org/wiki/Glibc
The GNU C Library, commonly known as glibc, is the C standard library released by the GNU Project. Originally written by the Free Software Foundation (FSF) for the GNU operating system, the library's development has been overseen by a committee since 2001,[2] with Ulrich Drepper from Red Hat as the lead contributor and maintainer.
Released under the GNU Lesser General Public License, glibc is free software.
B. uClibc
From: http://en.wikipedia.org/wiki/UClibc
In computing, uClibc is a small C standard library intended for embedded Linux systems. uClibc was created to support uClinux, a version of Linux not requiring a memory management unit and thus suited for microcontrollers (uCs; the "u" is a romanization of μ for "micro").[2]
Development on uClibc started around 1999.[3] uClibc was mostly written from scratch,[4] but has incorporated code from glibc and other projects.[5]
COMPILER LINKER TOOL CHAIN PATH IN LTIB
------------------------------------------------------
[q.yang@localhost ~]$ tree /opt/nxp/ -L 2
/opt/nxp/
`-- gcc-3.4.5-glibc-2.3.6
`-- arm-linux-gnu
[q.yang@localhost ~]$ tree /opt/freescale/ -L 4
/opt/freescale/
`-- usr
`-- local
`-- gcc-4.1.2-glibc-2.5-nptl-3
`-- arm-none-linux-gnueabi
[q.yang@localhost ~]$ tree CodeSourcery/ -L 3
CodeSourcery/
`-- arm-2010q1
|-- arm-none-linux-gnueabi
| |-- bin
| |-- include
| |-- lib
| |-- libc
| `-- share
|-- bin
| |-- arm-none-linux-gnueabi-addr2line
| |-- arm-none-linux-gnueabi-ar
| |-- arm-none-linux-gnueabi-as
| |-- arm-none-linux-gnueabi-c++
| |-- arm-none-linux-gnueabi-c++filt
| |-- arm-none-linux-gnueabi-cpp
| |-- arm-none-linux-gnueabi-g++
| |-- arm-none-linux-gnueabi-gcc
| |-- arm-none-linux-gnueabi-gcc-4.4.1
| |-- arm-none-linux-gnueabi-gcov
| |-- arm-none-linux-gnueabi-gdb
| |-- arm-none-linux-gnueabi-gdbtui
| |-- arm-none-linux-gnueabi-gprof
| |-- arm-none-linux-gnueabi-ld
| |-- arm-none-linux-gnueabi-nm
| |-- arm-none-linux-gnueabi-objcopy
| |-- arm-none-linux-gnueabi-objdump
| |-- arm-none-linux-gnueabi-ranlib
| |-- arm-none-linux-gnueabi-readelf
| |-- arm-none-linux-gnueabi-size
| |-- arm-none-linux-gnueabi-sprite
| |-- arm-none-linux-gnueabi-strings
| `-- arm-none-linux-gnueabi-strip
|-- lib
| `-- gcc
|-- libexec
| |-- arm-none-linux-gnueabi-post-install
| `-- gcc
`-- share
`-- doc
[q.yang@localhost ~]$ tree /opt/nxp/ -L 4
/opt/nxp/
`-- gcc-3.4.5-glibc-2.3.6
`-- arm-linux-gnu
|-- arm-linux-gnu
| |-- bin
| |-- etc
| |-- include
| |-- info
| |-- lib
| |-- libexec
| |-- share
| |-- sys-include
| `-- usr
|-- bin
| |-- arm-linux-gnu-addr2line
| |-- arm-linux-gnu-ar
| |-- arm-linux-gnu-as
| |-- arm-linux-gnu-c++
| |-- arm-linux-gnu-c++filt
| |-- arm-linux-gnu-cpp
| |-- arm-linux-gnu-g++
| |-- arm-linux-gnu-gcc
| |-- arm-linux-gnu-gcc-3.4.5
| |-- arm-linux-gnu-gccbug
| |-- arm-linux-gnu-gcov
| |-- arm-linux-gnu-gprof
| |-- arm-linux-gnu-ld
| |-- arm-linux-gnu-nm
| |-- arm-linux-gnu-objcopy
| |-- arm-linux-gnu-objdump
| |-- arm-linux-gnu-ranlib
| |-- arm-linux-gnu-readelf
| |-- arm-linux-gnu-size
| |-- arm-linux-gnu-strings
| |-- arm-linux-gnu-strip
| `-- fix-embedded-paths
|-- distributed
| |-- arm-linux-gnu -> ../arm-linux-gnu
| |-- bin
| |-- include -> ../include
| |-- info -> ../info
| |-- lib -> ../lib
| |-- libexec -> ../libexec
| `-- man -> ../man
|-- include
| `-- c++
|-- info
|-- lib
| |-- gcc
| `-- libiberty.a
|-- libexec
| `-- gcc
|-- man
| |-- man1
| `-- man7
`-- tmp
[q.yang@localhost ~]$ tree /opt/freescale/ -L 6
/opt/freescale/
`-- usr
`-- local
`-- gcc-4.1.2-glibc-2.5-nptl-3
`-- arm-none-linux-gnueabi
|-- arm-none-linux-gnueabi
| |-- bin
| |-- lib
| `-- sysroot
|-- bin
| |-- arm-none-linux-gnueabi-addr2line
| |-- arm-none-linux-gnueabi-ar
| |-- arm-none-linux-gnueabi-as
| |-- arm-none-linux-gnueabi-c++
| |-- arm-none-linux-gnueabi-c++filt
| |-- arm-none-linux-gnueabi-cpp
| |-- arm-none-linux-gnueabi-g++
| |-- arm-none-linux-gnueabi-gcc
| |-- arm-none-linux-gnueabi-gcc-4.1.2
| |-- arm-none-linux-gnueabi-gccbug
| |-- arm-none-linux-gnueabi-gcov
| |-- arm-none-linux-gnueabi-gprof
| |-- arm-none-linux-gnueabi-ld
| |-- arm-none-linux-gnueabi-nm
| |-- arm-none-linux-gnueabi-objcopy
| |-- arm-none-linux-gnueabi-objdump
| |-- arm-none-linux-gnueabi-ranlib
| |-- arm-none-linux-gnueabi-readelf
| |-- arm-none-linux-gnueabi-size
| |-- arm-none-linux-gnueabi-strings
| `-- arm-none-linux-gnueabi-strip
|-- include
| `-- c++
|-- info
|-- lib
| |-- gcc
| `-- libiberty.a
|-- libexec
| `-- gcc
|-- man
| |-- man1
| `-- man7
|-- share
`-- tmp
[2] GNU C Library - GLIBC
From libc.info and Coreutils.info
-----------------------------------
unlink,
rename,
if (stat("/dev/ttyUSB3",&buf)==0) {
//3G modem present
Misc::debug_print((GSN_S8*)"3G modem detected",GSN_LOG_INFO);
Misc::debug_print((GSN_S8*)"Killing pppd (if already running)",GSN_LOG_INFO);
if (system("killall pppd")==0) {
sleep(10);
GPIO_CMM_LNK_OFF;
}
rc = pthread_create(&pppthread, NULL, runpppd, &threadarg);
if (rc == 0) {
Misc::debug_print((GSN_S8*)"ppp thread created, sleeping to allow connection",GSN_LOG_INFO);
sleep(30);
} else {
Misc::debug_print((GSN_S8*)"failed to create ppp thread\n",GSN_LOG_INFO);
exit(1);
}
if (CommsLinkSessionMgr::isPPPConnectionAvailabe()) {
GPIO_CMM_LNK_ON;
} else {
GPIO_CMM_LNK_OFF;
}
}
1. Available Libraries.
-------------------------------------
A. Glibc
From: http://en.wikipedia.org/wiki/Glibc
The GNU C Library, commonly known as glibc, is the C standard library released by the GNU Project. Originally written by the Free Software Foundation (FSF) for the GNU operating system, the library's development has been overseen by a committee since 2001,[2] with Ulrich Drepper from Red Hat as the lead contributor and maintainer.
Released under the GNU Lesser General Public License, glibc is free software.
B. uClibc
From: http://en.wikipedia.org/wiki/UClibc
In computing, uClibc is a small C standard library intended for embedded Linux systems. uClibc was created to support uClinux, a version of Linux not requiring a memory management unit and thus suited for microcontrollers (uCs; the "u" is a romanization of μ for "micro").[2]
Development on uClibc started around 1999.[3] uClibc was mostly written from scratch,[4] but has incorporated code from glibc and other projects.[5]
COMPILER LINKER TOOL CHAIN PATH IN LTIB
------------------------------------------------------
[q.yang@localhost ~]$ tree /opt/nxp/ -L 2
/opt/nxp/
`-- gcc-3.4.5-glibc-2.3.6
`-- arm-linux-gnu
[q.yang@localhost ~]$ tree /opt/freescale/ -L 4
/opt/freescale/
`-- usr
`-- local
`-- gcc-4.1.2-glibc-2.5-nptl-3
`-- arm-none-linux-gnueabi
[q.yang@localhost ~]$ tree CodeSourcery/ -L 3
CodeSourcery/
`-- arm-2010q1
|-- arm-none-linux-gnueabi
| |-- bin
| |-- include
| |-- lib
| |-- libc
| `-- share
|-- bin
| |-- arm-none-linux-gnueabi-addr2line
| |-- arm-none-linux-gnueabi-ar
| |-- arm-none-linux-gnueabi-as
| |-- arm-none-linux-gnueabi-c++
| |-- arm-none-linux-gnueabi-c++filt
| |-- arm-none-linux-gnueabi-cpp
| |-- arm-none-linux-gnueabi-g++
| |-- arm-none-linux-gnueabi-gcc
| |-- arm-none-linux-gnueabi-gcc-4.4.1
| |-- arm-none-linux-gnueabi-gcov
| |-- arm-none-linux-gnueabi-gdb
| |-- arm-none-linux-gnueabi-gdbtui
| |-- arm-none-linux-gnueabi-gprof
| |-- arm-none-linux-gnueabi-ld
| |-- arm-none-linux-gnueabi-nm
| |-- arm-none-linux-gnueabi-objcopy
| |-- arm-none-linux-gnueabi-objdump
| |-- arm-none-linux-gnueabi-ranlib
| |-- arm-none-linux-gnueabi-readelf
| |-- arm-none-linux-gnueabi-size
| |-- arm-none-linux-gnueabi-sprite
| |-- arm-none-linux-gnueabi-strings
| `-- arm-none-linux-gnueabi-strip
|-- lib
| `-- gcc
|-- libexec
| |-- arm-none-linux-gnueabi-post-install
| `-- gcc
`-- share
`-- doc
[q.yang@localhost ~]$ tree /opt/nxp/ -L 4
/opt/nxp/
`-- gcc-3.4.5-glibc-2.3.6
`-- arm-linux-gnu
|-- arm-linux-gnu
| |-- bin
| |-- etc
| |-- include
| |-- info
| |-- lib
| |-- libexec
| |-- share
| |-- sys-include
| `-- usr
|-- bin
| |-- arm-linux-gnu-addr2line
| |-- arm-linux-gnu-ar
| |-- arm-linux-gnu-as
| |-- arm-linux-gnu-c++
| |-- arm-linux-gnu-c++filt
| |-- arm-linux-gnu-cpp
| |-- arm-linux-gnu-g++
| |-- arm-linux-gnu-gcc
| |-- arm-linux-gnu-gcc-3.4.5
| |-- arm-linux-gnu-gccbug
| |-- arm-linux-gnu-gcov
| |-- arm-linux-gnu-gprof
| |-- arm-linux-gnu-ld
| |-- arm-linux-gnu-nm
| |-- arm-linux-gnu-objcopy
| |-- arm-linux-gnu-objdump
| |-- arm-linux-gnu-ranlib
| |-- arm-linux-gnu-readelf
| |-- arm-linux-gnu-size
| |-- arm-linux-gnu-strings
| |-- arm-linux-gnu-strip
| `-- fix-embedded-paths
|-- distributed
| |-- arm-linux-gnu -> ../arm-linux-gnu
| |-- bin
| |-- include -> ../include
| |-- info -> ../info
| |-- lib -> ../lib
| |-- libexec -> ../libexec
| `-- man -> ../man
|-- include
| `-- c++
|-- info
|-- lib
| |-- gcc
| `-- libiberty.a
|-- libexec
| `-- gcc
|-- man
| |-- man1
| `-- man7
`-- tmp
[q.yang@localhost ~]$ tree /opt/freescale/ -L 6
/opt/freescale/
`-- usr
`-- local
`-- gcc-4.1.2-glibc-2.5-nptl-3
`-- arm-none-linux-gnueabi
|-- arm-none-linux-gnueabi
| |-- bin
| |-- lib
| `-- sysroot
|-- bin
| |-- arm-none-linux-gnueabi-addr2line
| |-- arm-none-linux-gnueabi-ar
| |-- arm-none-linux-gnueabi-as
| |-- arm-none-linux-gnueabi-c++
| |-- arm-none-linux-gnueabi-c++filt
| |-- arm-none-linux-gnueabi-cpp
| |-- arm-none-linux-gnueabi-g++
| |-- arm-none-linux-gnueabi-gcc
| |-- arm-none-linux-gnueabi-gcc-4.1.2
| |-- arm-none-linux-gnueabi-gccbug
| |-- arm-none-linux-gnueabi-gcov
| |-- arm-none-linux-gnueabi-gprof
| |-- arm-none-linux-gnueabi-ld
| |-- arm-none-linux-gnueabi-nm
| |-- arm-none-linux-gnueabi-objcopy
| |-- arm-none-linux-gnueabi-objdump
| |-- arm-none-linux-gnueabi-ranlib
| |-- arm-none-linux-gnueabi-readelf
| |-- arm-none-linux-gnueabi-size
| |-- arm-none-linux-gnueabi-strings
| `-- arm-none-linux-gnueabi-strip
|-- include
| `-- c++
|-- info
|-- lib
| |-- gcc
| `-- libiberty.a
|-- libexec
| `-- gcc
|-- man
| |-- man1
| `-- man7
|-- share
`-- tmp
Wednesday, July 21, 2010
Installation of CodeSourcery Tool Chain for ARM.
Check Available targets for current GDB installation
-------------------------------------------------------
[q.yang@localhost Download]$ gdb help target
GNU gdb Fedora (6.8-24.fc9)
Copyright (C) 2008 Free Software Foundation, Inc.
License GPLv3+: GNU GPL version 3 or later
This is free software: you are free to change and redistribute it.
There is NO WARRANTY, to the extent permitted by law. Type "show copying"
and "show warranty" for details.
This GDB was configured as "i386-redhat-linux-gnu"...
help: No such file or directory.
/home/q.yang/Download/target: No such file or directory.
(gdb) quit
INSTALL CODE SOURCERY COMPILER AND DEBUGGER
-------------------------------------------------------
[q.yang@localhost FromCodeSourcery]$ bunzip2 arm-2010q1-202-arm-none-linux-gnueabi-i686-pc-linux-gnu.tar.bz2
[q.yang@localhost FromCodeSourcery]$ mkdir -p ~/CodeSourcery
[q.yang@localhost FromCodeSourcery]$ cd ~/CodeSourcery/
[q.yang@localhost CodeSourcery]$ tar xf ~/Download/FromCodeSourcery/arm-2010q1-202-arm-none-linux-gnueabi-i686-pc-linux-gnu.tar
---Add $HOME/CodeSourcery/arm-2010q1/bin and ... into $PATH and $MANPATH------
[q.yang@localhost arm-2010q1]$ cat ~/.bash_profile
CODE_SRCERY_PATH=$HOME/CodeSourcery/arm-2010q1/bin
PATH=$PATH:$HOME/bin:/sbin:/usr/sbin:/opt/wx/2.8/bin:$LTIB_TOOL_CHAIN_FREESCALE_PATH:/opt/codeblocks20100721-svn/bin/:$CODE_SRCERY_PATH
MANPATH=$MANPATH:$HOME/CodeSourcery/arm-2010q1/share/doc/sourceryg++-arm-none-linux-gnueabi/man
VERIFY THE CODE SOURCERY TOOL CHAIN INSTALLATION
---------------------------------------------------
[q.yang@localhost arm-2010q1]$ arm-none-linux-gnueabi-g++ -v
Using built-in specs.
Target: arm-none-linux-gnueabi
Configured with: /scratch/julian/2010q1-release-linux-lite/src/gcc-4.4-2010q1/configure --build=i686-pc-linux-gnu --host=i686-pc-linux-gnu --target=arm-none-linux-gnueabi --enable-threads --disable-libmudflap --disable-libssp --disable-libstdcxx-pch --enable-extra-sgxxlite-multilibs --with-arch=armv5te --with-gnu-as --with-gnu-ld --with-specs='%{funwind-tables|fno-unwind-tables|mabi=*|ffreestanding|nostdlib:;:-funwind-tables} %{O2:%{!fno-remove-local-statics: -fremove-local-statics}} %{O*:%{O|O0|O1|O2|Os:;:%{!fno-remove-local-statics: -fremove-local-statics}}}' --enable-languages=c,c++ --enable-shared --disable-lto --enable-symvers=gnu --enable-__cxa_atexit --with-pkgversion='Sourcery G++ Lite 2010q1-202' --with-bugurl=https://support.codesourcery.com/GNUToolchain/ --disable-nls --prefix=/opt/codesourcery --with-sysroot=/opt/codesourcery/arm-none-linux-gnueabi/libc --with-build-sysroot=/scratch/julian/2010q1-release-linux-lite/install/arm-none-linux-gnueabi/libc --with-gmp=/scratch/julian/2010q1-release-linux-lite/obj/host-libs-2010q1-202-arm-none-linux-gnueabi-i686-pc-linux-gnu/usr --with-mpfr=/scratch/julian/2010q1-release-linux-lite/obj/host-libs-2010q1-202-arm-none-linux-gnueabi-i686-pc-linux-gnu/usr --with-ppl=/scratch/julian/2010q1-release-linux-lite/obj/host-libs-2010q1-202-arm-none-linux-gnueabi-i686-pc-linux-gnu/usr --with-host-libstdcxx='-static-libgcc -Wl,-Bstatic,-lstdc++,-Bdynamic -lm' --with-cloog=/scratch/julian/2010q1-release-linux-lite/obj/host-libs-2010q1-202-arm-none-linux-gnueabi-i686-pc-linux-gnu/usr --disable-libgomp --enable-poison-system-directories --with-build-time-tools=/scratch/julian/2010q1-release-linux-lite/install/arm-none-linux-gnueabi/bin --with-build-time-tools=/scratch/julian/2010q1-release-linux-lite/install/arm-none-linux-gnueabi/bin
Thread model: posix
gcc version 4.4.1 (Sourcery G++ Lite 2010q1-202)

CodeSourcery 32 bits version won't work under Fedora20 unless install glibc.i686 32bits glibc library.
[root@fedora20 q.yang]# yum install glibc.i686
[q.yang@fedora20 ~]$ arm-none-linux-gnueabi-g++ -v
Using built-in specs.
Target: arm-none-linux-gnueabi
Configured with: /scratch/julian/2010q1-release-linux-lite/src/gcc-4.4-2010q1/configure --build=i686-pc-linux-gnu --host=i686-pc-linux-gnu --target=arm-none-linux-gnueabi --enable-threads --disable-libmudflap --disable-libssp --disable-libstdcxx-pch --enable-extra-sgxxlite-multilibs --with-arch=armv5te --with-gnu-as --with-gnu-ld --with-specs='%{funwind-tables|fno-unwind-tables|mabi=*|ffreestanding|nostdlib:;:-funwind-tables} %{O2:%{!fno-remove-local-statics: -fremove-local-statics}} %{O*:%{O|O0|O1|O2|Os:;:%{!fno-remove-local-statics: -fremove-local-statics}}}' --enable-languages=c,c++ --enable-shared --disable-lto --enable-symvers=gnu --enable-__cxa_atexit --with-pkgversion='Sourcery G++ Lite 2010q1-202' --with-bugurl=https://support.codesourcery.com/GNUToolchain/ --disable-nls --prefix=/opt/codesourcery --with-sysroot=/opt/codesourcery/arm-none-linux-gnueabi/libc --with-build-sysroot=/scratch/julian/2010q1-release-linux-lite/install/arm-none-linux-gnueabi/libc --with-gmp=/scratch/julian/2010q1-release-linux-lite/obj/host-libs-2010q1-202-arm-none-linux-gnueabi-i686-pc-linux-gnu/usr --with-mpfr=/scratch/julian/2010q1-release-linux-lite/obj/host-libs-2010q1-202-arm-none-linux-gnueabi-i686-pc-linux-gnu/usr --with-ppl=/scratch/julian/2010q1-release-linux-lite/obj/host-libs-2010q1-202-arm-none-linux-gnueabi-i686-pc-linux-gnu/usr --with-host-libstdcxx='-static-libgcc -Wl,-Bstatic,-lstdc++,-Bdynamic -lm' --with-cloog=/scratch/julian/2010q1-release-linux-lite/obj/host-libs-2010q1-202-arm-none-linux-gnueabi-i686-pc-linux-gnu/usr --disable-libgomp --enable-poison-system-directories --with-build-time-tools=/scratch/julian/2010q1-release-linux-lite/install/arm-none-linux-gnueabi/bin --with-build-time-tools=/scratch/julian/2010q1-release-linux-lite/install/arm-none-linux-gnueabi/bin
Thread model: posix
gcc version 4.4.1 (Sourcery G++ Lite 2010q1-202)
Alternatively, download install latest 64bits CodeSouercery (called CodeBench ) from Mentor, it'll work with 64bits glibc library.
amd-2014.05-25-x86_64-amd-linux-gnu-i686-pc-linux-gnu.tar
-------------------------------------------------------
[q.yang@localhost Download]$ gdb help target
GNU gdb Fedora (6.8-24.fc9)
Copyright (C) 2008 Free Software Foundation, Inc.
License GPLv3+: GNU GPL version 3 or later
This is free software: you are free to change and redistribute it.
There is NO WARRANTY, to the extent permitted by law. Type "show copying"
and "show warranty" for details.
This GDB was configured as "i386-redhat-linux-gnu"...
help: No such file or directory.
/home/q.yang/Download/target: No such file or directory.
(gdb) quit
INSTALL CODE SOURCERY COMPILER AND DEBUGGER
-------------------------------------------------------
[q.yang@localhost FromCodeSourcery]$ bunzip2 arm-2010q1-202-arm-none-linux-gnueabi-i686-pc-linux-gnu.tar.bz2
[q.yang@localhost FromCodeSourcery]$ mkdir -p ~/CodeSourcery
[q.yang@localhost FromCodeSourcery]$ cd ~/CodeSourcery/
[q.yang@localhost CodeSourcery]$ tar xf ~/Download/FromCodeSourcery/arm-2010q1-202-arm-none-linux-gnueabi-i686-pc-linux-gnu.tar
---Add $HOME/CodeSourcery/arm-2010q1/bin and ... into $PATH and $MANPATH------
[q.yang@localhost arm-2010q1]$ cat ~/.bash_profile
CODE_SRCERY_PATH=$HOME/CodeSourcery/arm-2010q1/bin
PATH=$PATH:$HOME/bin:/sbin:/usr/sbin:/opt/wx/2.8/bin:$LTIB_TOOL_CHAIN_FREESCALE_PATH:/opt/codeblocks20100721-svn/bin/:$CODE_SRCERY_PATH
MANPATH=$MANPATH:$HOME/CodeSourcery/arm-2010q1/share/doc/sourceryg++-arm-none-linux-gnueabi/man
VERIFY THE CODE SOURCERY TOOL CHAIN INSTALLATION
---------------------------------------------------
[q.yang@localhost arm-2010q1]$ arm-none-linux-gnueabi-g++ -v
Using built-in specs.
Target: arm-none-linux-gnueabi
Configured with: /scratch/julian/2010q1-release-linux-lite/src/gcc-4.4-2010q1/configure --build=i686-pc-linux-gnu --host=i686-pc-linux-gnu --target=arm-none-linux-gnueabi --enable-threads --disable-libmudflap --disable-libssp --disable-libstdcxx-pch --enable-extra-sgxxlite-multilibs --with-arch=armv5te --with-gnu-as --with-gnu-ld --with-specs='%{funwind-tables|fno-unwind-tables|mabi=*|ffreestanding|nostdlib:;:-funwind-tables} %{O2:%{!fno-remove-local-statics: -fremove-local-statics}} %{O*:%{O|O0|O1|O2|Os:;:%{!fno-remove-local-statics: -fremove-local-statics}}}' --enable-languages=c,c++ --enable-shared --disable-lto --enable-symvers=gnu --enable-__cxa_atexit --with-pkgversion='Sourcery G++ Lite 2010q1-202' --with-bugurl=https://support.codesourcery.com/GNUToolchain/ --disable-nls --prefix=/opt/codesourcery --with-sysroot=/opt/codesourcery/arm-none-linux-gnueabi/libc --with-build-sysroot=/scratch/julian/2010q1-release-linux-lite/install/arm-none-linux-gnueabi/libc --with-gmp=/scratch/julian/2010q1-release-linux-lite/obj/host-libs-2010q1-202-arm-none-linux-gnueabi-i686-pc-linux-gnu/usr --with-mpfr=/scratch/julian/2010q1-release-linux-lite/obj/host-libs-2010q1-202-arm-none-linux-gnueabi-i686-pc-linux-gnu/usr --with-ppl=/scratch/julian/2010q1-release-linux-lite/obj/host-libs-2010q1-202-arm-none-linux-gnueabi-i686-pc-linux-gnu/usr --with-host-libstdcxx='-static-libgcc -Wl,-Bstatic,-lstdc++,-Bdynamic -lm' --with-cloog=/scratch/julian/2010q1-release-linux-lite/obj/host-libs-2010q1-202-arm-none-linux-gnueabi-i686-pc-linux-gnu/usr --disable-libgomp --enable-poison-system-directories --with-build-time-tools=/scratch/julian/2010q1-release-linux-lite/install/arm-none-linux-gnueabi/bin --with-build-time-tools=/scratch/julian/2010q1-release-linux-lite/install/arm-none-linux-gnueabi/bin
Thread model: posix
gcc version 4.4.1 (Sourcery G++ Lite 2010q1-202)
CodeSourcery 32 bits version won't work under Fedora20 unless install glibc.i686 32bits glibc library.
[root@fedora20 q.yang]# yum install glibc.i686
[q.yang@fedora20 ~]$ arm-none-linux-gnueabi-g++ -v
Using built-in specs.
Target: arm-none-linux-gnueabi
Configured with: /scratch/julian/2010q1-release-linux-lite/src/gcc-4.4-2010q1/configure --build=i686-pc-linux-gnu --host=i686-pc-linux-gnu --target=arm-none-linux-gnueabi --enable-threads --disable-libmudflap --disable-libssp --disable-libstdcxx-pch --enable-extra-sgxxlite-multilibs --with-arch=armv5te --with-gnu-as --with-gnu-ld --with-specs='%{funwind-tables|fno-unwind-tables|mabi=*|ffreestanding|nostdlib:;:-funwind-tables} %{O2:%{!fno-remove-local-statics: -fremove-local-statics}} %{O*:%{O|O0|O1|O2|Os:;:%{!fno-remove-local-statics: -fremove-local-statics}}}' --enable-languages=c,c++ --enable-shared --disable-lto --enable-symvers=gnu --enable-__cxa_atexit --with-pkgversion='Sourcery G++ Lite 2010q1-202' --with-bugurl=https://support.codesourcery.com/GNUToolchain/ --disable-nls --prefix=/opt/codesourcery --with-sysroot=/opt/codesourcery/arm-none-linux-gnueabi/libc --with-build-sysroot=/scratch/julian/2010q1-release-linux-lite/install/arm-none-linux-gnueabi/libc --with-gmp=/scratch/julian/2010q1-release-linux-lite/obj/host-libs-2010q1-202-arm-none-linux-gnueabi-i686-pc-linux-gnu/usr --with-mpfr=/scratch/julian/2010q1-release-linux-lite/obj/host-libs-2010q1-202-arm-none-linux-gnueabi-i686-pc-linux-gnu/usr --with-ppl=/scratch/julian/2010q1-release-linux-lite/obj/host-libs-2010q1-202-arm-none-linux-gnueabi-i686-pc-linux-gnu/usr --with-host-libstdcxx='-static-libgcc -Wl,-Bstatic,-lstdc++,-Bdynamic -lm' --with-cloog=/scratch/julian/2010q1-release-linux-lite/obj/host-libs-2010q1-202-arm-none-linux-gnueabi-i686-pc-linux-gnu/usr --disable-libgomp --enable-poison-system-directories --with-build-time-tools=/scratch/julian/2010q1-release-linux-lite/install/arm-none-linux-gnueabi/bin --with-build-time-tools=/scratch/julian/2010q1-release-linux-lite/install/arm-none-linux-gnueabi/bin
Thread model: posix
gcc version 4.4.1 (Sourcery G++ Lite 2010q1-202)
Alternatively, download install latest 64bits CodeSouercery (called CodeBench ) from Mentor, it'll work with 64bits glibc library.
amd-2014.05-25-x86_64-amd-linux-gnu-i686-pc-linux-gnu.tar
Firmware upgrade for Embedded Linux Device
http://www.embeddedrelated.com/usenet/embedded/show/21493-1.php
I devised the following system for an embedded linux system:
In flash I have:-
Backup Kernel
Backup Ramdisk
Operational Kernel
Operational Ramdisk
Bootloader
These images are compressed and checksummed.
When I load firmware, I buffer the image in RAM, verify the checksum, and
then copy over the operational firmware in flash. The Bootloader is never
field upgraded.
At boot time I set a flag in static RAM and count boot attempts. If a boot
fails the watchdog will reset the system. I cycle through each possible
combination of Kernel and Ramdisk [starting with the operational copies
and trying each combination 3 times if necessary].
When the system has booted, I start a little task that interrogates the
flags set by the bootloader, works out which images it booted from, compares
the checksum of the images it booted from with the images it didn't boot
from, and if they're different the image we booted from is copied over the
image we didn't boot from.
So far, I have never had a system fail to boot, despite power interruptions
during firmware upgrades and other fun things.
FROM EMBEDDED LINUX VIRTUAL CONFERENCE
----------------------------------------------
WanQiang(Quentin) YANG (10/22/2010 4:20:38 AM): Hi, what's the common way or is there common way to do firmware upgrade with embedded Linux? For our previous products without RTOS, firmware itself is able to reprogram the whole flash(ROM) with new firmware delievered via 3G modem.
Mike Anderson (10/22/2010 4:22:31 AM):@Wan: You could certainly do it that way. Boot firmware like UBoot could support that. But, I normally have a piece of firmware that I never allow the users to update so they can't brick the box. Then the O/S & filesystem are updated in flash via the boot firmware like you've done in the past
WanQiang(Quentin) YANG (10/22/2010 4:25:45 AM):Yeah, in our previous product, one piece of code, we call it bootloader, can never be upgraded, which stay same in a certain part of FLASH.
Raghavendra Reddy Desai (10/22/2010 4:28:20 AM):@ EROL :for cortex-M3 try using openICE EDS
WanQiang(Quentin) YANG (10/22/2010 4:28:40 AM):So, you reckon, I can do the same thing, get one level of boot loader before UBoot to manage the Embedded Linux upgrade for both Kernel and File System. Thanks. That'll be part of job outside of Linux Porting.
Mike Anderson (10/22/2010 4:29:25 AM):@Wan: Yes, that's what we've done many times in the past and that seems to work reliably. Good luck.
I devised the following system for an embedded linux system:
In flash I have:-
Backup Kernel
Backup Ramdisk
Operational Kernel
Operational Ramdisk
Bootloader
These images are compressed and checksummed.
When I load firmware, I buffer the image in RAM, verify the checksum, and
then copy over the operational firmware in flash. The Bootloader is never
field upgraded.
At boot time I set a flag in static RAM and count boot attempts. If a boot
fails the watchdog will reset the system. I cycle through each possible
combination of Kernel and Ramdisk [starting with the operational copies
and trying each combination 3 times if necessary].
When the system has booted, I start a little task that interrogates the
flags set by the bootloader, works out which images it booted from, compares
the checksum of the images it booted from with the images it didn't boot
from, and if they're different the image we booted from is copied over the
image we didn't boot from.
So far, I have never had a system fail to boot, despite power interruptions
during firmware upgrades and other fun things.
FROM EMBEDDED LINUX VIRTUAL CONFERENCE
----------------------------------------------
WanQiang(Quentin) YANG (10/22/2010 4:20:38 AM): Hi, what's the common way or is there common way to do firmware upgrade with embedded Linux? For our previous products without RTOS, firmware itself is able to reprogram the whole flash(ROM) with new firmware delievered via 3G modem.
Mike Anderson (10/22/2010 4:22:31 AM):@Wan: You could certainly do it that way. Boot firmware like UBoot could support that. But, I normally have a piece of firmware that I never allow the users to update so they can't brick the box. Then the O/S & filesystem are updated in flash via the boot firmware like you've done in the past
WanQiang(Quentin) YANG (10/22/2010 4:25:45 AM):Yeah, in our previous product, one piece of code, we call it bootloader, can never be upgraded, which stay same in a certain part of FLASH.
Raghavendra Reddy Desai (10/22/2010 4:28:20 AM):@ EROL :for cortex-M3 try using openICE EDS
WanQiang(Quentin) YANG (10/22/2010 4:28:40 AM):So, you reckon, I can do the same thing, get one level of boot loader before UBoot to manage the Embedded Linux upgrade for both Kernel and File System. Thanks. That'll be part of job outside of Linux Porting.
Mike Anderson (10/22/2010 4:29:25 AM):@Wan: Yes, that's what we've done many times in the past and that seems to work reliably. Good luck.
Tuesday, July 20, 2010
Install Code::Blocks in Fedora 9.
Ref [1] http://wiki.codeblocks.org/index.php?title=Installing_Code::Blocks_from_source_on_Linux
Ref [2] http://www.friendlyarm.net/forum/topic/26
1.
-------------------------------
[q.yang@localhost ~]$ mkdir ~/devel
2.Checking the presence of GTK+ library
---------------------------------------
[q.yang@localhost ~]$ rpm -qa |grep gtk
pygtk2-codegen-2.12.1-6.fc9.i386
gtk-nodoka-engine-0.7.1-2.fc9.i386
gtk-doc-1.9-4.fc9.noarch
authconfig-gtk-5.4.4-1.fc9.i386
gtkmm24-2.12.7-1.fc9.i386
gtksourceview2-2.2.2-1.fc9.i386
gtkhtml2-2.11.1-3.fc9.i386
pygtk2-doc-2.12.1-6.fc9.i386
pygtksourceview-2.2.0-1.fc9.i386
usermode-gtk-1.98-1.fc9.i386
gtk2-engines-2.14.3-1.fc9.i386
gnome-python2-gtkhtml2-2.19.1-28.fc9.i386
gtk2-2.12.12-2.fc9.i386
gtkspell-2.0.11-8.fc9.i386
gtk-sharp2-2.12.1-1.fc9.i386
GConf2-gtk-2.22.0-1.fc9.i386
pygtk2-libglade-2.12.1-6.fc9.i386
pygtk2-2.12.1-6.fc9.i386
xdg-user-dirs-gtk-0.7-1.fc9.i386
pygtk2-devel-2.12.1-6.fc9.i386
gtkhtml3-3.18.3-1.fc9.i386
gtk2-devel-2.12.12-2.fc9.i386
[q.yang@localhost ~]$ ll /usr/lib/ | grep libgtk
lrwxrwxrwx 1 root root 21 2010-06-04 02:47 libgtkhtml-2.so.0 -> libgtkhtml-2.so.0.0.0
-rwxr-xr-x 1 root root 451304 2008-02-10 14:34 libgtkhtml-2.so.0.0.0
lrwxrwxrwx 1 root root 25 2010-06-07 18:04 libgtkhtml-3.14.so.19 -> libgtkhtml-3.14.so.19.1.0
-rwxr-xr-x 1 root root 764296 2008-06-30 21:58 libgtkhtml-3.14.so.19.1.0
lrwxrwxrwx 1 root root 22 2010-06-07 17:50 libgtkmm-2.4.so.1 -> libgtkmm-2.4.so.1.0.30
-rwxr-xr-x 1 root root 3446444 2008-04-12 22:43 libgtkmm-2.4.so.1.0.30
-rwxr-xr-x 1 root root 24160 2008-09-20 19:23 libgtksharpglue-2.so
lrwxrwxrwx 1 root root 29 2010-06-07 17:50 libgtksourceview-2.0.so.0 -> libgtksourceview-2.0.so.0.0.0
-rwxr-xr-x 1 root root 264908 2008-07-02 01:00 libgtksourceview-2.0.so.0.0.0
lrwxrwxrwx 1 root root 20 2010-06-04 02:36 libgtkspell.so.0 -> libgtkspell.so.0.0.0
-rwxr-xr-x 1 root root 21540 2008-02-10 14:29 libgtkspell.so.0.0.0
lrwxrwxrwx 1 root root 27 2010-06-07 18:23 libgtk-x11-2.0.so -> libgtk-x11-2.0.so.0.1200.12
lrwxrwxrwx 1 root root 27 2010-06-07 17:47 libgtk-x11-2.0.so.0 -> libgtk-x11-2.0.so.0.1200.12
-rwxr-xr-x 1 root root 4233032 2008-11-21 19:06 libgtk-x11-2.0.so.0.1200.12
3. Checking the presence of libwxGTK library
---------------------------------------
A. Download source if not installed or no yum rpm package available.
[q.yang@localhost ~]$ cd devel/
[q.yang@localhost devel]$ ll
-rw-rw-r-- 1 q.yang q.yang 13078779 2010-07-21 16:27 wxGTK-2.8.11.tar.gz
[q.yang@localhost devel]$ tar zxf wxGTK-2.8.11.tar.gz
[q.yang@localhost devel]$ cd wxGTK-2.8.11
B. Config, compile and install.
[q.yang@localhost wxGTK-2.8.11]$ mkdir build_gtk2_shared_monolithic_unicode
[q.yang@localhost wxGTK-2.8.11]$ cd build_gtk2_shared_monolithic_unicode/
[q.yang@localhost build_gtk2_shared_monolithic_unicode]$ ../configure --prefix=/opt/wx/2.8 \
> --enable-xrc \
> --enable-monolithic \
> --enable-unicode
......................
..................
config.status: creating contrib/src/net/Makefile
config.status: creating lib/wx/include/gtk2-unicode-release-2.8/wx/setup.h
config.status: executing wx-config commands
Configured wxWidgets 2.8.11 for `i686-pc-linux-gnu'
Which GUI toolkit should wxWidgets use? GTK+ 2 with support for gnomeprint
Should wxWidgets be compiled into single library? yes
Should wxWidgets be compiled in debug mode? no
Should wxWidgets be linked as a shared library? yes
Should wxWidgets be compiled in Unicode mode? yes
What level of wxWidgets compatibility should be enabled?
wxWidgets 2.4 no
wxWidgets 2.6 yes
Which libraries should wxWidgets use?
jpeg sys
png sys
regex builtin
tiff sys
zlib sys
odbc no
expat sys
libmspack no
sdl no
[q.yang@localhost build_gtk2_shared_monolithic_unicode]$ make
[root@localhost build_gtk2_shared_monolithic_unicode]# make install
...................................................................
...................................................................
if test ! -d /opt/wx/2.8/include/wx-2.8/`dirname $f` ; then \
/usr/bin/install -c -d /opt/wx/2.8/include/wx-2.8/`dirname $f`; \
fi; \
/usr/bin/install -c -m 644 ../include/$f /opt/wx/2.8/include/wx-2.8/$f; \
done
------------------------------------------------------
The installation of wxWidgets is finished. On certain
platforms (e.g. Linux) you'll now have to run ldconfig
if you installed a shared library and also modify the
LD_LIBRARY_PATH (or equivalent) environment variable.
wxWidgets comes with no guarantees and doesn't claim
to be suitable for any purpose.
Read the wxWidgets Licence on licencing conditions.
------------------------------------------------------
C. Added /opt/wx/2.8/bin into $PATH
[q.yang@localhost build_gtk2_shared_monolithic_unicode]$ vi ~/.bash_profile
[q.yang@localhost build_gtk2_shared_monolithic_unicode]$ source ~/.bash_profile
[q.yang@localhost build_gtk2_shared_monolithic_unicode]$ echo $PATH
/usr/lib/qt-3.3/bin:/usr/kerberos/bin:/usr/lib/ccache:/usr/local/bin:/bin:/usr/bin:/home/q.yang/bin:/sbin:/usr/sbin:/home/q.yang/j2sdk1.4.2_12/bin:/home/q.yang/j2sdk1.4.2_12/jre/bin:/home/q.yang/eclipse_workspace/MyMacProj/bin:/home/q.yang/bin:/sbin:/usr/sbin:/opt/wx/2.8/bin:/home/q.yang/j2sdk1.4.2_12/bin:/home/q.yang/j2sdk1.4.2_12/jre/bin:/home/q.yang/eclipse_workspace/MyMacProj/bin
D. Some extra changes.
[root@localhost build_gtk2_shared_monolithic_unicode]# vi /etc/ld.so.conf
[root@localhost build_gtk2_shared_monolithic_unicode]# exit
exit
[q.yang@localhost build_gtk2_shared_monolithic_unicode]$ cat /etc/ld.so.conf
include ld.so.conf.d/*.conf
include /opt/wx/2.8/lib
[root@localhost build_gtk2_shared_monolithic_unicode]# ldconfig
[q.yang@localhost build_gtk2_shared_monolithic_unicode]$ wx-config --prefix
/opt/wx/2.8
[q.yang@localhost build_gtk2_shared_monolithic_unicode]$ wx-config --libs
-L/opt/wx/2.8/lib -pthread -lwx_gtk2u-2.8
[q.yang@localhost build_gtk2_shared_monolithic_unicode]$ which wx-config
/opt/wx/2.8/bin/wx-config
4. Getting Code::Blocks sources
---------------------------------------------------------------
[q.yang@localhost devel]$ svn checkout svn://svn.berlios.de/codeblocks/trunk
.....................................
...................................
A trunk/TODO
A trunk/codeblocks.spec.in
A trunk/acinclude.m4
A trunk/codeblocks.plist.in
A trunk/COPYING
A trunk/Makefile.am
A trunk/update_revision.sh
A trunk/NEWS
A trunk/bootstrap
A trunk/codeblocks.pc.in
A trunk/README.debian
Checked out revision 6411.
[q.yang@localhost trunk]$ ./bootstrap
...............................
configure.in:77: error: possibly undefined macro: AM_OPTIONS_WXCONFIG
If this token and others are legitimate, please use m4_pattern_allow.
See the Autoconf documentation.
configure.in:78: error: possibly undefined macro: AM_PATH_WXCONFIG
[root@localhost trunk]# echo `wx-config --prefix`/share/aclocal >> /usr/share/aclocal/dirlist
[q.yang@localhost trunk]$ ./bootstrap THIS TIME IT'LL HAVE NO ERROR.
5. Install code:block
---------------------------------------
[q.yang@localhost trunk]$ ./configure ALL INSTALLATION WILL BE USING DEFAULT SETTING.
[q.yang@localhost trunk]$./configure --prefix=/opt/codeblocks20100721-svn --with-contrib-plugins=all
......................................
config.status: creating src/include/config.h
config.status: src/include/config.h is unchanged
config.status: executing depfiles commands
*************************************************
* Code::Blocks source tree has been configured. *
*************************************************
You can now build Code::Blocks by issuing 'make'.
When the build is complete, become root and install
it by issuing 'make install'.
[q.yang@localhost trunk]$ make
........error occurred during make ..... in particular in /plug-in/...
[root@localhost trunk]# make install
.............................
PATH="$PATH:/sbin" ldconfig -n /opt/codeblocks20100721-svn/lib/wxSmithContribItems
----------------------------------------------------------------------
Libraries have been installed in:
/opt/codeblocks20100721-svn/lib/wxSmithContribItems
If you ever happen to want to link against installed libraries
in a given directory, LIBDIR, you must either use libtool, and
specify the full pathname of the library, or use the `-LLIBDIR'
flag during linking and do at least one of the following:
- add LIBDIR to the `LD_LIBRARY_PATH' environment variable
during execution
- add LIBDIR to the `LD_RUN_PATH' environment variable
during linking
- use the `-Wl,--rpath -Wl,LIBDIR' linker flag
- have your system administrator add LIBDIR to `/etc/ld.so.conf'
See any operating system documentation about shared libraries for
more information, such as the ld(1) and ld.so(8) manual pages.
----------------------------------------------------------------------
....................................
CParser.cpp:111: error: 'preprocessor' was not declared in this scope
CParser.cpp:115: error: 'comment' was not declared in this scope
CParser.cpp:119: error: 'special_w' was not declared in this scope
CParser.cpp:145: error: 'parse_info' was not declared in this scope
CParser.cpp:145: error: expected primary-expression before 'const'
CParser.cpp:145: error: expected `;' before 'const'
CParser.cpp:151: error: 'info' was not declared in this scope
make[4]: *** [CParser.lo] Error 1
make[4]: Leaving directory `/home/q.yang/devel/trunk/src/plugins/contrib/NassiShneiderman'
make[3]: *** [install-recursive] Error 1
make[3]: Leaving directory `/home/q.yang/devel/trunk/src/plugins/contrib'
make[2]: *** [install-recursive] Error 1
make[2]: Leaving directory `/home/q.yang/devel/trunk/src/plugins'
make[1]: *** [install-recursive] Error 1
make[1]: Leaving directory `/home/q.yang/devel/trunk/src'
make: *** [install-recursive] Error 1
6. Last thing to do before running code:blocks
-------------------------------------------------------
[q.yang@localhost trunk]$ /opt/codeblocks20100721-svn/bin/codeblocks
/opt/codeblocks20100721-svn/bin/codeblocks: error while loading shared libraries: libwx_gtk2u-2.8.so.0: cannot open shared object file: No such file or directory
[root@localhost trunk]# vi /etc/ld.so.conf
[root@localhost trunk]# ldconfig
[q.yang@localhost trunk]$ cat /etc/ld.so.conf
include ld.so.conf.d/*.conf
/opt/wx/2.8/lib
/opt/codeblocks20100721-svn/lib
7. DONE ---- Code::blocks can be started now
--------------------------------------------------
[q.yang@localhost trunk]$ /opt/codeblocks20100721-svn/bin/codeblocks
Initialize EditColourSet .....
Initialize EditColourSet: done.
8. Set Compiler to use LTIB arm tool Chain
----------------------------------------------
Code:Blocks svn build rev 6411
1. navigate to home/username and edit .bash_profile
LTIB_TOOL_CHAIN_FREESCALE_PATH=/opt/freescale/usr/local/gcc-4.1.2-glibc-2.5-nptl-3/arm-none-linux-gnueabi
PATH=$PATH:$HOME/bin:/sbin:/usr/sbin:/opt/wx/2.8/bin:$LTIB_TOOL_CHAIN_FREESCALE_PATH:/opt/codeblocks20100721-svn/bin/
2. $source .bash_profile
3. Go to Settings/Compiler and debugger and in Select compiler find GNU
ARM GCC compiler, select it and Set as default or it reverts to GNU GCC
Compiler everytime you start a new project
4. in the tab Toolchain executables (make sure this window is maximised or
you don’t see this tab!) and Compiler’s installation directory put
/opt/freescale/usr/local/gcc-4.1.2-glibc-2.5-nptl-3/arm-none-linux-gnueabi (bin not necessary)
5. now in the Program Files window:
manually select and map the c/c++ compiler, linker with actual 'commands'
C compiler arm-none-linux-gnueabi-gcc-4.1.2
C++ compiler arm-none-linux-gnueabi-g++
Linker for dynamic libs arm-none-linux-gnueabi-gcc-g++
Linker for static libs arm-none-linux-gnueabi-ar
Debugger gdb (Make sure [*] cross gdb (runs on build machine) ticked in LTIB, so that u can find gdb in ~/lpc3250/ltib-qs/bin/ )
make program make
Then add /usr/bin/ into addtional path so that compiler can find where is 'make' command.

Successfully passed GDB debugging after adding and enabling [*]cross gdb Tool Chain in LTIB, and configured it in Code blocks(/ltib-qs/bin/gdb). Here's the screen shot when using LTIB tool chain for debugging.

Successfully passed GDB debugging after intalling CodeSourcery Tool Chain, and configured it in Code blocks. Here's the screen shot when using code sourcery.
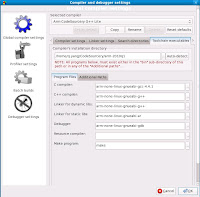
To make it easy to identify, you can rename the compiler
Ref [2] http://www.friendlyarm.net/forum/topic/26
1.
-------------------------------
[q.yang@localhost ~]$ mkdir ~/devel
2.Checking the presence of GTK+ library
---------------------------------------
[q.yang@localhost ~]$ rpm -qa |grep gtk
pygtk2-codegen-2.12.1-6.fc9.i386
gtk-nodoka-engine-0.7.1-2.fc9.i386
gtk-doc-1.9-4.fc9.noarch
authconfig-gtk-5.4.4-1.fc9.i386
gtkmm24-2.12.7-1.fc9.i386
gtksourceview2-2.2.2-1.fc9.i386
gtkhtml2-2.11.1-3.fc9.i386
pygtk2-doc-2.12.1-6.fc9.i386
pygtksourceview-2.2.0-1.fc9.i386
usermode-gtk-1.98-1.fc9.i386
gtk2-engines-2.14.3-1.fc9.i386
gnome-python2-gtkhtml2-2.19.1-28.fc9.i386
gtk2-2.12.12-2.fc9.i386
gtkspell-2.0.11-8.fc9.i386
gtk-sharp2-2.12.1-1.fc9.i386
GConf2-gtk-2.22.0-1.fc9.i386
pygtk2-libglade-2.12.1-6.fc9.i386
pygtk2-2.12.1-6.fc9.i386
xdg-user-dirs-gtk-0.7-1.fc9.i386
pygtk2-devel-2.12.1-6.fc9.i386
gtkhtml3-3.18.3-1.fc9.i386
gtk2-devel-2.12.12-2.fc9.i386
[q.yang@localhost ~]$ ll /usr/lib/ | grep libgtk
lrwxrwxrwx 1 root root 21 2010-06-04 02:47 libgtkhtml-2.so.0 -> libgtkhtml-2.so.0.0.0
-rwxr-xr-x 1 root root 451304 2008-02-10 14:34 libgtkhtml-2.so.0.0.0
lrwxrwxrwx 1 root root 25 2010-06-07 18:04 libgtkhtml-3.14.so.19 -> libgtkhtml-3.14.so.19.1.0
-rwxr-xr-x 1 root root 764296 2008-06-30 21:58 libgtkhtml-3.14.so.19.1.0
lrwxrwxrwx 1 root root 22 2010-06-07 17:50 libgtkmm-2.4.so.1 -> libgtkmm-2.4.so.1.0.30
-rwxr-xr-x 1 root root 3446444 2008-04-12 22:43 libgtkmm-2.4.so.1.0.30
-rwxr-xr-x 1 root root 24160 2008-09-20 19:23 libgtksharpglue-2.so
lrwxrwxrwx 1 root root 29 2010-06-07 17:50 libgtksourceview-2.0.so.0 -> libgtksourceview-2.0.so.0.0.0
-rwxr-xr-x 1 root root 264908 2008-07-02 01:00 libgtksourceview-2.0.so.0.0.0
lrwxrwxrwx 1 root root 20 2010-06-04 02:36 libgtkspell.so.0 -> libgtkspell.so.0.0.0
-rwxr-xr-x 1 root root 21540 2008-02-10 14:29 libgtkspell.so.0.0.0
lrwxrwxrwx 1 root root 27 2010-06-07 18:23 libgtk-x11-2.0.so -> libgtk-x11-2.0.so.0.1200.12
lrwxrwxrwx 1 root root 27 2010-06-07 17:47 libgtk-x11-2.0.so.0 -> libgtk-x11-2.0.so.0.1200.12
-rwxr-xr-x 1 root root 4233032 2008-11-21 19:06 libgtk-x11-2.0.so.0.1200.12
3. Checking the presence of libwxGTK library
---------------------------------------
A. Download source if not installed or no yum rpm package available.
[q.yang@localhost ~]$ cd devel/
[q.yang@localhost devel]$ ll
-rw-rw-r-- 1 q.yang q.yang 13078779 2010-07-21 16:27 wxGTK-2.8.11.tar.gz
[q.yang@localhost devel]$ tar zxf wxGTK-2.8.11.tar.gz
[q.yang@localhost devel]$ cd wxGTK-2.8.11
B. Config, compile and install.
[q.yang@localhost wxGTK-2.8.11]$ mkdir build_gtk2_shared_monolithic_unicode
[q.yang@localhost wxGTK-2.8.11]$ cd build_gtk2_shared_monolithic_unicode/
[q.yang@localhost build_gtk2_shared_monolithic_unicode]$ ../configure --prefix=/opt/wx/2.8 \
> --enable-xrc \
> --enable-monolithic \
> --enable-unicode
......................
..................
config.status: creating contrib/src/net/Makefile
config.status: creating lib/wx/include/gtk2-unicode-release-2.8/wx/setup.h
config.status: executing wx-config commands
Configured wxWidgets 2.8.11 for `i686-pc-linux-gnu'
Which GUI toolkit should wxWidgets use? GTK+ 2 with support for gnomeprint
Should wxWidgets be compiled into single library? yes
Should wxWidgets be compiled in debug mode? no
Should wxWidgets be linked as a shared library? yes
Should wxWidgets be compiled in Unicode mode? yes
What level of wxWidgets compatibility should be enabled?
wxWidgets 2.4 no
wxWidgets 2.6 yes
Which libraries should wxWidgets use?
jpeg sys
png sys
regex builtin
tiff sys
zlib sys
odbc no
expat sys
libmspack no
sdl no
[q.yang@localhost build_gtk2_shared_monolithic_unicode]$ make
[root@localhost build_gtk2_shared_monolithic_unicode]# make install
...................................................................
...................................................................
if test ! -d /opt/wx/2.8/include/wx-2.8/`dirname $f` ; then \
/usr/bin/install -c -d /opt/wx/2.8/include/wx-2.8/`dirname $f`; \
fi; \
/usr/bin/install -c -m 644 ../include/$f /opt/wx/2.8/include/wx-2.8/$f; \
done
------------------------------------------------------
The installation of wxWidgets is finished. On certain
platforms (e.g. Linux) you'll now have to run ldconfig
if you installed a shared library and also modify the
LD_LIBRARY_PATH (or equivalent) environment variable.
wxWidgets comes with no guarantees and doesn't claim
to be suitable for any purpose.
Read the wxWidgets Licence on licencing conditions.
------------------------------------------------------
C. Added /opt/wx/2.8/bin into $PATH
[q.yang@localhost build_gtk2_shared_monolithic_unicode]$ vi ~/.bash_profile
[q.yang@localhost build_gtk2_shared_monolithic_unicode]$ source ~/.bash_profile
[q.yang@localhost build_gtk2_shared_monolithic_unicode]$ echo $PATH
/usr/lib/qt-3.3/bin:/usr/kerberos/bin:/usr/lib/ccache:/usr/local/bin:/bin:/usr/bin:/home/q.yang/bin:/sbin:/usr/sbin:/home/q.yang/j2sdk1.4.2_12/bin:/home/q.yang/j2sdk1.4.2_12/jre/bin:/home/q.yang/eclipse_workspace/MyMacProj/bin:/home/q.yang/bin:/sbin:/usr/sbin:/opt/wx/2.8/bin:/home/q.yang/j2sdk1.4.2_12/bin:/home/q.yang/j2sdk1.4.2_12/jre/bin:/home/q.yang/eclipse_workspace/MyMacProj/bin
D. Some extra changes.
[root@localhost build_gtk2_shared_monolithic_unicode]# vi /etc/ld.so.conf
[root@localhost build_gtk2_shared_monolithic_unicode]# exit
exit
[q.yang@localhost build_gtk2_shared_monolithic_unicode]$ cat /etc/ld.so.conf
include ld.so.conf.d/*.conf
include /opt/wx/2.8/lib
[root@localhost build_gtk2_shared_monolithic_unicode]# ldconfig
[q.yang@localhost build_gtk2_shared_monolithic_unicode]$ wx-config --prefix
/opt/wx/2.8
[q.yang@localhost build_gtk2_shared_monolithic_unicode]$ wx-config --libs
-L/opt/wx/2.8/lib -pthread -lwx_gtk2u-2.8
[q.yang@localhost build_gtk2_shared_monolithic_unicode]$ which wx-config
/opt/wx/2.8/bin/wx-config
4. Getting Code::Blocks sources
---------------------------------------------------------------
[q.yang@localhost devel]$ svn checkout svn://svn.berlios.de/codeblocks/trunk
.....................................
...................................
A trunk/TODO
A trunk/codeblocks.spec.in
A trunk/acinclude.m4
A trunk/codeblocks.plist.in
A trunk/COPYING
A trunk/Makefile.am
A trunk/update_revision.sh
A trunk/NEWS
A trunk/bootstrap
A trunk/codeblocks.pc.in
A trunk/README.debian
Checked out revision 6411.
[q.yang@localhost trunk]$ ./bootstrap
...............................
configure.in:77: error: possibly undefined macro: AM_OPTIONS_WXCONFIG
If this token and others are legitimate, please use m4_pattern_allow.
See the Autoconf documentation.
configure.in:78: error: possibly undefined macro: AM_PATH_WXCONFIG
[root@localhost trunk]# echo `wx-config --prefix`/share/aclocal >> /usr/share/aclocal/dirlist
[q.yang@localhost trunk]$ ./bootstrap THIS TIME IT'LL HAVE NO ERROR.
5. Install code:block
---------------------------------------
[q.yang@localhost trunk]$ ./configure ALL INSTALLATION WILL BE USING DEFAULT SETTING.
[q.yang@localhost trunk]$./configure --prefix=/opt/codeblocks20100721-svn --with-contrib-plugins=all
......................................
config.status: creating src/include/config.h
config.status: src/include/config.h is unchanged
config.status: executing depfiles commands
*************************************************
* Code::Blocks source tree has been configured. *
*************************************************
You can now build Code::Blocks by issuing 'make'.
When the build is complete, become root and install
it by issuing 'make install'.
[q.yang@localhost trunk]$ make
........error occurred during make ..... in particular in /plug-in/...
[root@localhost trunk]# make install
.............................
PATH="$PATH:/sbin" ldconfig -n /opt/codeblocks20100721-svn/lib/wxSmithContribItems
----------------------------------------------------------------------
Libraries have been installed in:
/opt/codeblocks20100721-svn/lib/wxSmithContribItems
If you ever happen to want to link against installed libraries
in a given directory, LIBDIR, you must either use libtool, and
specify the full pathname of the library, or use the `-LLIBDIR'
flag during linking and do at least one of the following:
- add LIBDIR to the `LD_LIBRARY_PATH' environment variable
during execution
- add LIBDIR to the `LD_RUN_PATH' environment variable
during linking
- use the `-Wl,--rpath -Wl,LIBDIR' linker flag
- have your system administrator add LIBDIR to `/etc/ld.so.conf'
See any operating system documentation about shared libraries for
more information, such as the ld(1) and ld.so(8) manual pages.
----------------------------------------------------------------------
....................................
CParser.cpp:111: error: 'preprocessor' was not declared in this scope
CParser.cpp:115: error: 'comment' was not declared in this scope
CParser.cpp:119: error: 'special_w' was not declared in this scope
CParser.cpp:145: error: 'parse_info' was not declared in this scope
CParser.cpp:145: error: expected primary-expression before 'const'
CParser.cpp:145: error: expected `;' before 'const'
CParser.cpp:151: error: 'info' was not declared in this scope
make[4]: *** [CParser.lo] Error 1
make[4]: Leaving directory `/home/q.yang/devel/trunk/src/plugins/contrib/NassiShneiderman'
make[3]: *** [install-recursive] Error 1
make[3]: Leaving directory `/home/q.yang/devel/trunk/src/plugins/contrib'
make[2]: *** [install-recursive] Error 1
make[2]: Leaving directory `/home/q.yang/devel/trunk/src/plugins'
make[1]: *** [install-recursive] Error 1
make[1]: Leaving directory `/home/q.yang/devel/trunk/src'
make: *** [install-recursive] Error 1
6. Last thing to do before running code:blocks
-------------------------------------------------------
[q.yang@localhost trunk]$ /opt/codeblocks20100721-svn/bin/codeblocks
/opt/codeblocks20100721-svn/bin/codeblocks: error while loading shared libraries: libwx_gtk2u-2.8.so.0: cannot open shared object file: No such file or directory
[root@localhost trunk]# vi /etc/ld.so.conf
[root@localhost trunk]# ldconfig
[q.yang@localhost trunk]$ cat /etc/ld.so.conf
include ld.so.conf.d/*.conf
/opt/wx/2.8/lib
/opt/codeblocks20100721-svn/lib
7. DONE ---- Code::blocks can be started now
--------------------------------------------------
[q.yang@localhost trunk]$ /opt/codeblocks20100721-svn/bin/codeblocks
Initialize EditColourSet .....
Initialize EditColourSet: done.
8. Set Compiler to use LTIB arm tool Chain
----------------------------------------------
Code:Blocks svn build rev 6411
1. navigate to home/username and edit .bash_profile
LTIB_TOOL_CHAIN_FREESCALE_PATH=/opt/freescale/usr/local/gcc-4.1.2-glibc-2.5-nptl-3/arm-none-linux-gnueabi
PATH=$PATH:$HOME/bin:/sbin:/usr/sbin:/opt/wx/2.8/bin:$LTIB_TOOL_CHAIN_FREESCALE_PATH:/opt/codeblocks20100721-svn/bin/
2. $source .bash_profile
3. Go to Settings/Compiler and debugger and in Select compiler find GNU
ARM GCC compiler, select it and Set as default or it reverts to GNU GCC
Compiler everytime you start a new project
4. in the tab Toolchain executables (make sure this window is maximised or
you don’t see this tab!) and Compiler’s installation directory put
/opt/freescale/usr/local/gcc-4.1.2-glibc-2.5-nptl-3/arm-none-linux-gnueabi (bin not necessary)
5. now in the Program Files window:
manually select and map the c/c++ compiler, linker with actual 'commands'
C compiler arm-none-linux-gnueabi-gcc-4.1.2
C++ compiler arm-none-linux-gnueabi-g++
Linker for dynamic libs arm-none-linux-gnueabi-gcc-g++
Linker for static libs arm-none-linux-gnueabi-ar
Debugger gdb (Make sure [*] cross gdb (runs on build machine) ticked in LTIB, so that u can find gdb in ~/lpc3250/ltib-qs/bin/ )
make program make
Then add /usr/bin/ into addtional path so that compiler can find where is 'make' command.
Successfully passed GDB debugging after adding and enabling [*]cross gdb Tool Chain in LTIB, and configured it in Code blocks(/ltib-qs/bin/gdb). Here's the screen shot when using LTIB tool chain for debugging.
Successfully passed GDB debugging after intalling CodeSourcery Tool Chain, and configured it in Code blocks. Here's the screen shot when using code sourcery.
To make it easy to identify, you can rename the compiler
FW: Six Popular IDEs For Developing Software in C/C++ on Windows Platform
http://beans.seartipy.com/2006/12/31/six-popular-ides-for-developing-software-in-cc-on-windows-platform/
Six Popular IDEs For Developing Software in C/C++ on Windows Platform
Six Popular IDEs For Developing Software in C/C++ on Windows Platform
Sunday, July 18, 2010
MOVE /HOME DIRECTORY TO 2ND HARD DISK
MOVE /HOME DIRECTORY TO 2ND HARD DISK
-----------------------------------------------
#mkdir -p /mnt/200GData
#mount /dev/sdb5 /mnt/200GData
#cp -rpf /home/* /mnt/200GData transfer current home to 2nd hard drive
#umount /mnt/200GData
#vi /etc/fstab add two lines /dev/sdb5, /dev/sdb1
[root@localhost ltib-qs]# cat /etc/fstab
/dev/VolGroup00/LogVol00 / ext3 defaults 1 1
UUID=db85ce6b-b0e2-4f47-ad07-011f425bd92c /boot ext3 defaults 1 2
tmpfs /dev/shm tmpfs defaults 0 0
devpts /dev/pts devpts gid=5,mode=620 0 0
sysfs /sys sysfs defaults 0 0
proc /proc proc defaults 0 0
/dev/VolGroup00/LogVol01 swap swap defaults 0 0
/dev/sdb5 /home ext2 defaults 0 0
/dev/sdb1 /opt ext2 defaults 0 0
#mount -a
Now the /home is on 2nd drive /dev/sdb5, if #umount /home, /home is on original 1st drive.
Ref [1] http://forums.fedoraforum.org/showthread.php?t=179402
[2] http://www.ibm.com/developerworks/library/l-partplan.html
SELinx warning after move /home to seperate hard drive
----------------------------------------------------------------
SELinux permission checks on files labeled file_t are being denied. file_t is
the context the SELinux kernel gives to files that do not have a label. This
indicates a serious labeling problem. No files on an SELinux box should ever be
labeled file_t. If you have just added a new disk drive to the system you can
relabel it using the restorecon command. Otherwise you should relabel the entire
files system.
[root@localhost /]# restorecon -r /home/
restorecon: unable to stat file /home/q.yang/.gvfs: Permission denied
[root@localhost /]# restorecon -r /opt/
-----------------------------------------------
#mkdir -p /mnt/200GData
#mount /dev/sdb5 /mnt/200GData
#cp -rpf /home/* /mnt/200GData transfer current home to 2nd hard drive
#umount /mnt/200GData
#vi /etc/fstab add two lines /dev/sdb5, /dev/sdb1
[root@localhost ltib-qs]# cat /etc/fstab
/dev/VolGroup00/LogVol00 / ext3 defaults 1 1
UUID=db85ce6b-b0e2-4f47-ad07-011f425bd92c /boot ext3 defaults 1 2
tmpfs /dev/shm tmpfs defaults 0 0
devpts /dev/pts devpts gid=5,mode=620 0 0
sysfs /sys sysfs defaults 0 0
proc /proc proc defaults 0 0
/dev/VolGroup00/LogVol01 swap swap defaults 0 0
/dev/sdb5 /home ext2 defaults 0 0
/dev/sdb1 /opt ext2 defaults 0 0
#mount -a
Now the /home is on 2nd drive /dev/sdb5, if #umount /home, /home is on original 1st drive.
Ref [1] http://forums.fedoraforum.org/showthread.php?t=179402
[2] http://www.ibm.com/developerworks/library/l-partplan.html
SELinx warning after move /home to seperate hard drive
----------------------------------------------------------------
SELinux permission checks on files labeled file_t are being denied. file_t is
the context the SELinux kernel gives to files that do not have a label. This
indicates a serious labeling problem. No files on an SELinux box should ever be
labeled file_t. If you have just added a new disk drive to the system you can
relabel it using the restorecon command. Otherwise you should relabel the entire
files system.
[root@localhost /]# restorecon -r /home/
restorecon: unable to stat file /home/q.yang/.gvfs: Permission denied
[root@localhost /]# restorecon -r /opt/
Wednesday, July 14, 2010
Build Linux Kernel from Source in Fedora 9
Ref [1] http://fedoraproject.org/wiki/Docs/CustomKernel#Configure_Kernel_Options
Ref [2] http://www.howtoforge.com/kernel_compilation_fedora
GET KERNEL SOURCE CODE BY EITHER YUM OR DIRECTLY FROM HTTP SITE
--------------------------------------------------------------
WHERE IS KERNEL SOURCE CODE IN FEDORA 9
----------------------------------------------------
JUST GET HEADER FILES OF KERNEL (enough to compile some extra modules)
-----------------------------------------------------------------------
kernel-devel-2.6.25-14.fc9.i686.rpm only gives you the header files of linux kernel
[root@localhost sierra_drv_1.7.32]# yum install kernel-devel-2.6.25-14.fc9.i686
DIRECT DOWNLOAD KERNEL RPM
--------------------------------
cd /usr/src
wget http://download.fedora.redhat.com/pub/fedora/linux/core/9/source/SRPMS/kernel-2.6.25-14.fc9.src.rpm
rpm -ivh kernel-2.6.18-1.2798.fc6.src.rpm
NOTE: .src.rpm is no longer availabe in above path.
GET KERNEL RPM VIA YUM
-----------------------------------
yum install rpmdevtools yum-utils
yumdownloader --destdir /home/q.yang/Download/LinuxSrcRpm2.6.27/ --source kernel
[q.yang@localhost ~]$ rpmdev-setuptree
[q.yang@localhost ~]$ su -c 'yum-builddep Download/LinuxSrcRpm2.6.27/kernel-2.6.27.25-78.2.56.fc9.src.rpm'
[q.yang@localhost ~]$ rpm -ivh /home/q.yang/Download/LinuxSrcRpm2.6.27/kernel-2.6.27.25-78.2.56.fc9.src.rpm
[q.yang@localhost SPECS]$ rpmbuild -bp --target=i686 kernel.spec
BUILD KERNEL ON USER SPACE
-----------------------------
1. Prepare a RPM package building environment in your home directory. Run the following command:
[q.yang@localhost ~]$ rpmdev-setuptree
[q.yang@localhost ~]$ ll
total 40
drwxr-xr-x 2 q.yang q.yang 4096 2010-06-03 17:23 Desktop
drwxr-xr-x 4 q.yang q.yang 4096 2010-07-06 10:54 Documents
drwxr-xr-x 4 q.yang q.yang 4096 2010-07-06 09:54 Download
drwxrwxr-x 3 q.yang q.yang 4096 2010-06-10 11:37 lpc3250
drwxr-xr-x 2 q.yang q.yang 4096 2010-06-03 17:13 Music
drwxr-xr-x 2 q.yang q.yang 4096 2010-06-03 17:13 Pictures
drwxr-xr-x 2 q.yang q.yang 4096 2010-06-03 17:13 Public
drwxrwxr-x 7 q.yang q.yang 4096 2010-07-06 10:55 rpmbuild NEW DIRECTORY
drwxr-xr-x 2 q.yang q.yang 4096 2010-06-03 17:13 Templates
drwxr-xr-x 2 q.yang q.yang 4096 2010-06-03 17:13 Videos
rpmbuild directory automatically only locate in user's home directory (i.e., ~)
and a new .rpmmacros will appear
NOTE: pls run 'rpmdev-setuptree' command with q.yang user instead of root. It will impact where the target directory of 'rpm -ivh ....' command.
2. Install build dependencies for the kernel source with the yum-builddep command (root is required to install these packages):
[q.yang@localhost ~]$ su -c 'yum-builddep Download/LinuxSrcRpm2.6.27/kernel-2.6.27.25-78.2.56.fc9.src.rpm'
Password:
Loaded plugins: refresh-packagekit
module-init-tools-3.4-13.fc9.i386
patch-2.5.4-35.fc9.i386
bash-3.2-23.fc9.i386
coreutils-6.10-35.fc9.i386
2:tar-1.19-6.fc9.i386
bzip2-1.0.5-2.fc9.i386
1:findutils-4.2.33-3.fc9.i386
gzip-1.3.12-6.fc9.i386
m4-1.4.10-3.fc9.i386
4:perl-5.10.0-68.fc9.i386
1:make-3.81-12.fc9.i386
diffutils-2.8.1-21.fc9.i386
gawk-3.1.5-17.fc9.i386
gcc-4.3.0-8.i386
binutils-2.18.50.0.6-7.fc9.i386
redhat-rpm-config-9.0.2-1.fc9.noarch
rpm-build-4.4.2.3-3.fc9.i386
No uninstalled build requires
3. Install kernel-.src.rpm with the following command
rpm -ivh /home/q.yang/Download/LinuxSrcRpm2.6.27/kernel-2.6.27.25-78.2.56.fc9.src.rpm
New installation use:
[q.yang@localhost ~]$ rpm -ivh /home/q.yang/Download/LinuxSrcRpm2.6.27/kernel-2.6.27.25-78.2.56.fc9.src.rpm
To just update the installation use:
[q.yang@localhost ~]$ rpm -Uvh Download/LinuxSrcRpm2.6.27/kernel-2.6.27.25-78.2.56.fc9.src.rpm
1:kernel warning: user mockbuild does not exist - using root
warning: group mockbuild does not exist - using root
warning: user mockbuild does not exist - using root
warning: group mockbuild does not exist - using root
( Afterwards, new files appear under path : /home/q.yang/rpmbuild/SOURCES/ )
4. This step expands all of the source code files for the kernel. This is required to view the code, edit the code, or to generate a patch.
Prepare the kernel source tree using the following commands:
[q.yang@localhost SPECS]$ rpmbuild -bp --target=i686 kernel.spec
(
Afterwards, you will see all source codes and new directories under path /BUILD/:
|-- BUILD
| `-- kernel-2.6.27
| |-- linux-2.6.27.i686
| `-- vanilla-2.6.27
vanilla-2.6.27/drivers/rtc/rtc-sysfs.c
..........................
vanilla-2.6.27/drivers/rtc/rtc-bfin.c
................................
vanilla-2.6.27/drivers/rtc/rtc-sh.c
vanilla-2.6.27/drivers/watchdog/alim1535_wdt.c
....................................
vanilla-2.6.27/drivers/watchdog/pcwd_pci.c
vanilla-2.6.27/drivers/watchdog/s3c2410_wdt.c
linux-2.6.27.i686/drivers/rtc/rtc-sysfs.c
..........................
linux-2.6.27.i686/drivers/rtc/rtc-bfin.c
................................
linux-2.6.27.i686/drivers/rtc/rtc-sh.c
linux-2.6.27.i686/drivers/watchdog/alim1535_wdt.c
....................................
linux-2.6.27.i686/drivers/watchdog/pcwd_pci.c
linux-2.6.27.i686/drivers/watchdog/s3c2410_wdt.c
5. Do a source code copy if need to generate kernel patch.
[q.yang@localhost ~]$ cp -r ~/rpmbuild/BUILD/kernel-2.6.27/linux-2.6.27.i686 ~/rpmbuild/BUILD/kernel-2.6.27.orig
[q.yang@localhost ~]$ cp -al ~/rpmbuild/BUILD/kernel-2.6.27.orig/ ~/rpmbuild/BUILD/kernel-2.6.27.new
6. Config customized kernel.
[q.yang@localhost linux-2.6.27.i686]$ pwd
/home/q.yang/rpmbuild/BUILD/kernel-2.6.27/linux-2.6.27.i686
[q.yang@localhost linux-2.6.27.i686]$ make menuconfig
HOSTCC scripts/kconfig/lxdialog/checklist.o
HOSTCC scripts/kconfig/lxdialog/inputbox.o
HOSTCC scripts/kconfig/lxdialog/menubox.o
HOSTCC scripts/kconfig/lxdialog/textbox.o
HOSTCC scripts/kconfig/lxdialog/util.o
HOSTCC scripts/kconfig/lxdialog/yesno.o
HOSTCC scripts/kconfig/mconf.o
HOSTLD scripts/kconfig/mconf
scripts/kconfig/mconf arch/x86/Kconfig
#
# configuration written to .config
#
*** End of Linux kernel configuration.
*** Execute 'make' to build the kernel or try 'make help'.
7. Edit .config and copy to /rpmbuild/SOURCE
Add a new line to the top of the config file that contains the hardware platform the kernel is built for (the output of uname -i).
[q.yang@localhost linux-2.6.27.i686]$ vi .config
Added '# i386' in the first line of .config
[q.yang@localhost linux-2.6.27.i686]$ cp .config ~/rpmbuild/SOURCES/config-i686
===Following steps will Prepare Build Files======
8. Edit /rpmbuild/SPECS/kernel.spec
[q.yang@localhost linux-2.6.27.i686]$ cd ~/rpmbuild/SPECS/
[q.yang@localhost SPECS]$ vi kernel.spec
%define buildid .gsncommsdev
9. If you generated a patch, add the patch to the kernel.spec file, preferably at the end of all the existing patches and clearly commented.
The patch then needs to be applied in the patch application section of the spec file. Again, at the end of the existing patch applications and clearly commented.
===Following steps will build the new kernel=============
10.
To build all kernel flavors:
[q.yang@localhost SPECS]$ rpmbuild -bb --target=i686 kernel.spec
= To disable specific kernel flavors from the build (for a faster build):
rpmbuild -bb --without
Ref [2] http://www.howtoforge.com/kernel_compilation_fedora
GET KERNEL SOURCE CODE BY EITHER YUM OR DIRECTLY FROM HTTP SITE
--------------------------------------------------------------
WHERE IS KERNEL SOURCE CODE IN FEDORA 9
----------------------------------------------------
JUST GET HEADER FILES OF KERNEL (enough to compile some extra modules)
-----------------------------------------------------------------------
kernel-devel-2.6.25-14.fc9.i686.rpm only gives you the header files of linux kernel
[root@localhost sierra_drv_1.7.32]# yum install kernel-devel-2.6.25-14.fc9.i686
DIRECT DOWNLOAD KERNEL RPM
--------------------------------
cd /usr/src
wget http://download.fedora.redhat.com/pub/fedora/linux/core/9/source/SRPMS/kernel-2.6.25-14.fc9.src.rpm
rpm -ivh kernel-2.6.18-1.2798.fc6.src.rpm
NOTE: .src.rpm is no longer availabe in above path.
GET KERNEL RPM VIA YUM
-----------------------------------
yum install rpmdevtools yum-utils
yumdownloader --destdir /home/q.yang/Download/LinuxSrcRpm2.6.27/ --source kernel
[q.yang@localhost ~]$ rpmdev-setuptree
[q.yang@localhost ~]$ su -c 'yum-builddep Download/LinuxSrcRpm2.6.27/kernel-2.6.27.25-78.2.56.fc9.src.rpm'
[q.yang@localhost ~]$ rpm -ivh /home/q.yang/Download/LinuxSrcRpm2.6.27/kernel-2.6.27.25-78.2.56.fc9.src.rpm
[q.yang@localhost SPECS]$ rpmbuild -bp --target=i686 kernel.spec
BUILD KERNEL ON USER SPACE
-----------------------------
1. Prepare a RPM package building environment in your home directory. Run the following command:
[q.yang@localhost ~]$ rpmdev-setuptree
[q.yang@localhost ~]$ ll
total 40
drwxr-xr-x 2 q.yang q.yang 4096 2010-06-03 17:23 Desktop
drwxr-xr-x 4 q.yang q.yang 4096 2010-07-06 10:54 Documents
drwxr-xr-x 4 q.yang q.yang 4096 2010-07-06 09:54 Download
drwxrwxr-x 3 q.yang q.yang 4096 2010-06-10 11:37 lpc3250
drwxr-xr-x 2 q.yang q.yang 4096 2010-06-03 17:13 Music
drwxr-xr-x 2 q.yang q.yang 4096 2010-06-03 17:13 Pictures
drwxr-xr-x 2 q.yang q.yang 4096 2010-06-03 17:13 Public
drwxrwxr-x 7 q.yang q.yang 4096 2010-07-06 10:55 rpmbuild NEW DIRECTORY
drwxr-xr-x 2 q.yang q.yang 4096 2010-06-03 17:13 Templates
drwxr-xr-x 2 q.yang q.yang 4096 2010-06-03 17:13 Videos
rpmbuild directory automatically only locate in user's home directory (i.e., ~)
and a new .rpmmacros will appear
NOTE: pls run 'rpmdev-setuptree' command with q.yang user instead of root. It will impact where the target directory of 'rpm -ivh ....' command.
2. Install build dependencies for the kernel source with the yum-builddep command (root is required to install these packages):
[q.yang@localhost ~]$ su -c 'yum-builddep Download/LinuxSrcRpm2.6.27/kernel-2.6.27.25-78.2.56.fc9.src.rpm'
Password:
Loaded plugins: refresh-packagekit
module-init-tools-3.4-13.fc9.i386
patch-2.5.4-35.fc9.i386
bash-3.2-23.fc9.i386
coreutils-6.10-35.fc9.i386
2:tar-1.19-6.fc9.i386
bzip2-1.0.5-2.fc9.i386
1:findutils-4.2.33-3.fc9.i386
gzip-1.3.12-6.fc9.i386
m4-1.4.10-3.fc9.i386
4:perl-5.10.0-68.fc9.i386
1:make-3.81-12.fc9.i386
diffutils-2.8.1-21.fc9.i386
gawk-3.1.5-17.fc9.i386
gcc-4.3.0-8.i386
binutils-2.18.50.0.6-7.fc9.i386
redhat-rpm-config-9.0.2-1.fc9.noarch
rpm-build-4.4.2.3-3.fc9.i386
No uninstalled build requires
3. Install kernel-
rpm -ivh /home/q.yang/Download/LinuxSrcRpm2.6.27/kernel-2.6.27.25-78.2.56.fc9.src.rpm
New installation use:
[q.yang@localhost ~]$ rpm -ivh /home/q.yang/Download/LinuxSrcRpm2.6.27/kernel-2.6.27.25-78.2.56.fc9.src.rpm
To just update the installation use:
[q.yang@localhost ~]$ rpm -Uvh Download/LinuxSrcRpm2.6.27/kernel-2.6.27.25-78.2.56.fc9.src.rpm
1:kernel warning: user mockbuild does not exist - using root
warning: group mockbuild does not exist - using root
warning: user mockbuild does not exist - using root
warning: group mockbuild does not exist - using root
( Afterwards, new files appear under path : /home/q.yang/rpmbuild/SOURCES/ )
4. This step expands all of the source code files for the kernel. This is required to view the code, edit the code, or to generate a patch.
Prepare the kernel source tree using the following commands:
[q.yang@localhost SPECS]$ rpmbuild -bp --target=i686 kernel.spec
(
Afterwards, you will see all source codes and new directories under path /BUILD/:
|-- BUILD
| `-- kernel-2.6.27
| |-- linux-2.6.27.i686
| `-- vanilla-2.6.27
vanilla-2.6.27/drivers/rtc/rtc-sysfs.c
..........................
vanilla-2.6.27/drivers/rtc/rtc-bfin.c
................................
vanilla-2.6.27/drivers/rtc/rtc-sh.c
vanilla-2.6.27/drivers/watchdog/alim1535_wdt.c
....................................
vanilla-2.6.27/drivers/watchdog/pcwd_pci.c
vanilla-2.6.27/drivers/watchdog/s3c2410_wdt.c
linux-2.6.27.i686/drivers/rtc/rtc-sysfs.c
..........................
linux-2.6.27.i686/drivers/rtc/rtc-bfin.c
................................
linux-2.6.27.i686/drivers/rtc/rtc-sh.c
linux-2.6.27.i686/drivers/watchdog/alim1535_wdt.c
....................................
linux-2.6.27.i686/drivers/watchdog/pcwd_pci.c
linux-2.6.27.i686/drivers/watchdog/s3c2410_wdt.c
5. Do a source code copy if need to generate kernel patch.
[q.yang@localhost ~]$ cp -r ~/rpmbuild/BUILD/kernel-2.6.27/linux-2.6.27.i686 ~/rpmbuild/BUILD/kernel-2.6.27.orig
[q.yang@localhost ~]$ cp -al ~/rpmbuild/BUILD/kernel-2.6.27.orig/ ~/rpmbuild/BUILD/kernel-2.6.27.new
6. Config customized kernel.
[q.yang@localhost linux-2.6.27.i686]$ pwd
/home/q.yang/rpmbuild/BUILD/kernel-2.6.27/linux-2.6.27.i686
[q.yang@localhost linux-2.6.27.i686]$ make menuconfig
HOSTCC scripts/kconfig/lxdialog/checklist.o
HOSTCC scripts/kconfig/lxdialog/inputbox.o
HOSTCC scripts/kconfig/lxdialog/menubox.o
HOSTCC scripts/kconfig/lxdialog/textbox.o
HOSTCC scripts/kconfig/lxdialog/util.o
HOSTCC scripts/kconfig/lxdialog/yesno.o
HOSTCC scripts/kconfig/mconf.o
HOSTLD scripts/kconfig/mconf
scripts/kconfig/mconf arch/x86/Kconfig
#
# configuration written to .config
#
*** End of Linux kernel configuration.
*** Execute 'make' to build the kernel or try 'make help'.
7. Edit .config and copy to /rpmbuild/SOURCE
Add a new line to the top of the config file that contains the hardware platform the kernel is built for (the output of uname -i).
[q.yang@localhost linux-2.6.27.i686]$ vi .config
Added '# i386' in the first line of .config
[q.yang@localhost linux-2.6.27.i686]$ cp .config ~/rpmbuild/SOURCES/config-i686
===Following steps will Prepare Build Files======
8. Edit /rpmbuild/SPECS/kernel.spec
[q.yang@localhost linux-2.6.27.i686]$ cd ~/rpmbuild/SPECS/
[q.yang@localhost SPECS]$ vi kernel.spec
%define buildid .gsncommsdev
9. If you generated a patch, add the patch to the kernel.spec file, preferably at the end of all the existing patches and clearly commented.
The patch then needs to be applied in the patch application section of the spec file. Again, at the end of the existing patch applications and clearly commented.
===Following steps will build the new kernel=============
10.
To build all kernel flavors:
[q.yang@localhost SPECS]$ rpmbuild -bb --target=i686 kernel.spec
= To disable specific kernel flavors from the build (for a faster build):
rpmbuild -bb --without
Subscribe to:
Posts (Atom)